To rename a file in Java, use the renameTo method of the File class. We will show you the code for its execution
package com.geekole; // Name of your java package
import java.io.File;
/**
*
* @author geekole.com
*/
public class RenameFile {
public static void main(String[] args) {
File oldfile = new File("/path/file.txt");
File newfile = new File("/path/newFile.txt");
if (oldfile.renameTo(newfile)) {
System.out.println("Renamed file");
} else {
System.out.println("error");
}
}
}
package com.geekole; // Name of your java package
import java.io.File;
/**
*
* @author geekole.com
*/
public class RenameFile {
public static void main(String[] args) {
File oldfile = new File("/path/file.txt");
File newfile = new File("/path/newFile.txt");
if (oldfile.renameTo(newfile)) {
System.out.println("Renamed file");
} else {
System.out.println("error");
}
}
}
package com.geekole; // Name of your java package import java.io.File; /** * * @author geekole.com */ public class RenameFile { public static void main(String[] args) { File oldfile = new File("/path/file.txt"); File newfile = new File("/path/newFile.txt"); if (oldfile.renameTo(newfile)) { System.out.println("Renamed file"); } else { System.out.println("error"); } } }
Of course, in your example you must take care that the new and old file paths are valid and that you have read and write permissions.
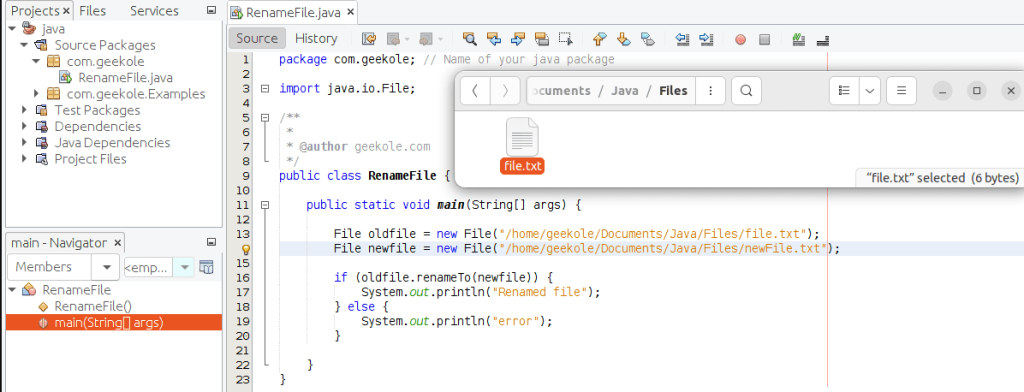
And that will be it, if the file does not exist or an error occurs while renaming the renameTo function will return false.
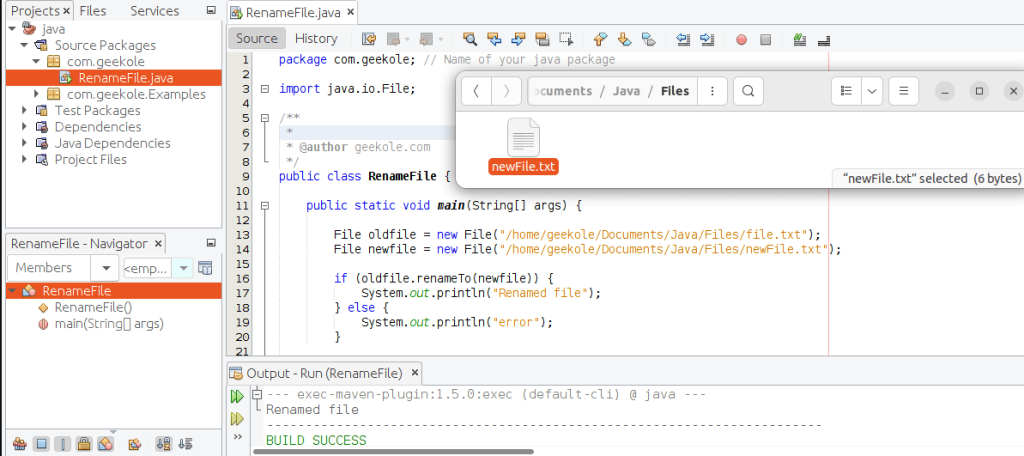
Something important that you may have noticed is that when you rename the file with the renameTo function you can change a file’s directory or folder, simply by setting a different path before the name.
We hope that the example of Rename a file in Java will be useful to you.