Here we explain the different Data Types in Java, with some simple examples to understand each one:
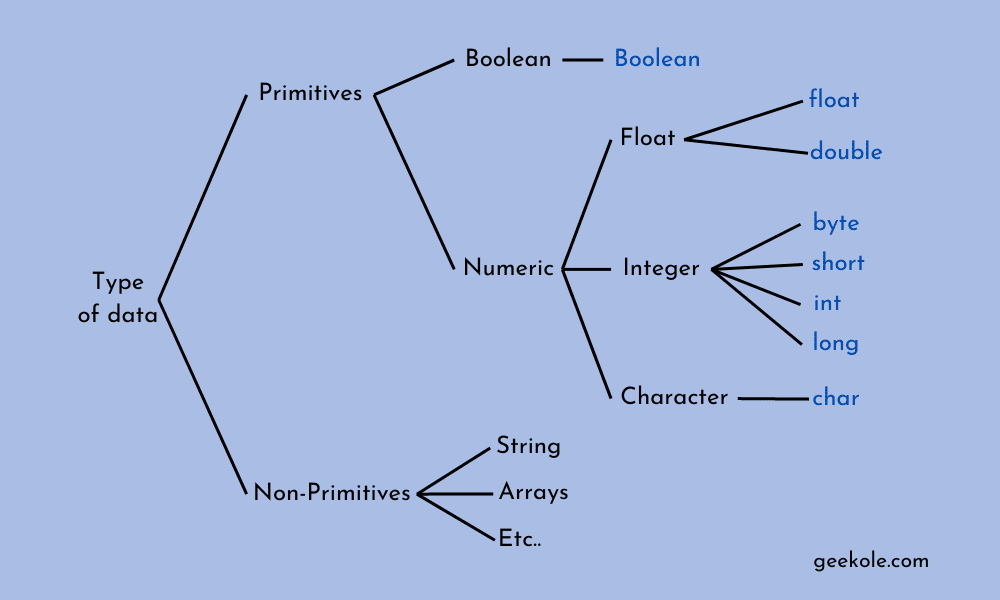
Primitive data types
The primitive data types that exist in Java are the following:
- byte
- shorts
- int
- long
- float
- double
- boolean
- char
Integers
Integer types (byte, short, int, and long) can contain integers such as 10 and −50. The size of the values that can be stored depends on the type of integer that is chosen.
Type | Size | Range of values that can be stored |
---|---|---|
byte | 1 byte | −128 to 127 |
short | 2 bytes | −32768 to 32767 |
int | 4 bytes | −2,147,483,648 to 2,147,483,647 |
long | 8 bytes | 9,223,372,036,854,775,808 to 9,223,372,036,854,755,807 |
//Examples of integer types: byte variableByte = 10; short variableShort = 266; int variableShort = 1231232; long variableLong = 1000000000l; //Notice the letter L at the end
Floating point numbers
Floating-point data types are used to represent numbers with a fractional part. Single-precision floating-point numbers are 4 bytes long, and double-precision floating-point numbers are 8 bytes long.
Type | Size | Range of values that can be stored |
---|---|---|
float | 4 bytes | 3.4e−038 to 3.4e+038 |
double | 8 bytes | 1.7e−308 to 1.7e+038 |
//Examples of floating point numbers float floatingNumber = 4.15f; double variableDouble = 12312.2;
Booleans
Boolean data types are used to store values with two states: true or false.
//Boolean example boolean exists = true;
Characters
The char data type stores characters. It assumes a size of 2 bytes, but it can only hold one character because char stores Unicode character sets. It has a minimum value of “u0000” (or 0) and a maximum value of “uffff” (or 65,535).
//Char example char c = 'a'; char b = '\n'; //Line break character
Non-Primitive Data Types
Character strings
A String is always enclosed in double quotes and is implemented using the java.lang.String class. Enclosing a string in double quotes will automatically create a new String object. For example:
//Strings example String cad = "This is a chain";
String objects are immutable, which means that once created, their values cannot be changed.
Other non-primitive data types are all those classes such as Arrays, Lists, etc.