We show you how to do Base64 Encoding and Decoding in Java with the new Java 8 APIs. What do you need this for? since making binary information is represented in Base64 is especially useful when it is required to send or store information as text. For example, when a Web Service receives a binary file as a parameter, it is usually sent and received by the service as a String in order to form a valid SOAP request, although it is not the only situation where this conversion may be required.
In Java 8 Base64 capabilities have finally been added to the standard API, via the java.util.Base64 utility class, as this was resolved with third-party libraries and has now been integrated as part of the standard Java 8 utility package. Java.
The following code block shows how to do it:
package com.geekole; // Name of your java package import java.util.Base64; /** * @author geekole.com */ public class ExampleBase64EncodingDecoding { public static void main(String args[]){ String originalEntry = "Test text."; String encodedString = Base64.getEncoder().encodeToString(originalEntry.getBytes()); System.out.println("encoded: " + encodedString); byte[] decodedBytes = Base64.getDecoder().decode(encodedString); String decodedString = new String(decodedBytes); System.out.println("decoded: " + decodedString); } }
In the first part you will base64 a string with the help of the encodeToString method. The string encoded in the variable “encodedString” is decoded using the decode method.
If the code is executed you will get something like the following:
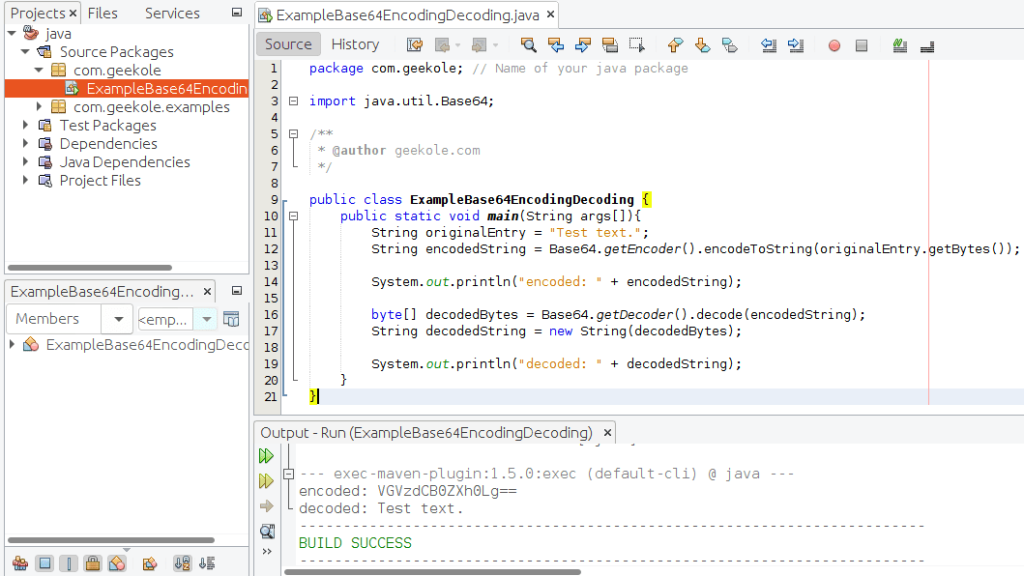
It’s quite easy to do Base64 Encoding and Decoding in Java, we hope you find this example useful.
Note: the examples are edited and run in NetBeans.
Official documentation: https://docs.oracle.com/javase/8/docs/api/java/util/Base64.html