We will work with the use of break and continue within the for and while loops in java in a simple and clear way.
Break
The use of the break statement allows a for or while loop to be interrupted and execution to jump out of the block of code. For example in the following sentences:
package com.geekole; // Name of your java package public class BreakExample { public static void main(String args[]){ for (int i = 0; i < 10; i++) { if(i == 6) { break; } System.out.println("i: " + i); } } }
If you run this code you get a sequence of numbers that stops when i equals 6 and doesn’t print any more.
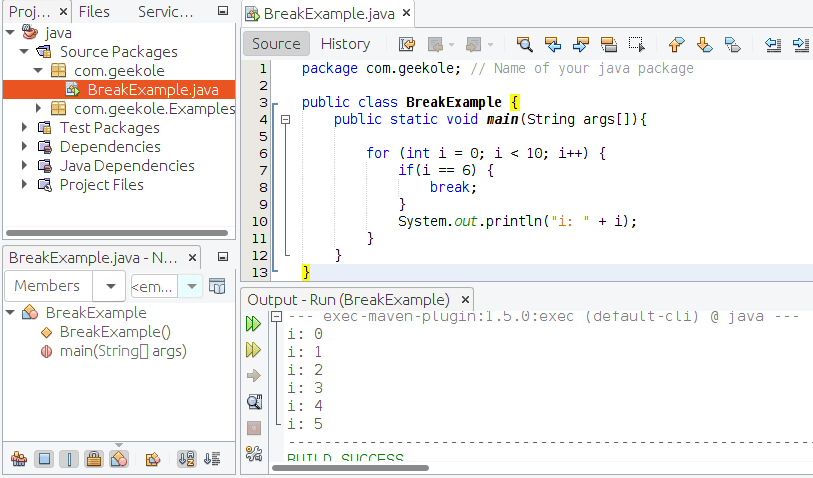
Similarly for a while loop, execution is interrupted.
package com.geekole; // Name of your java package public class BreakWhileExample { public static void main(String args[]){ int i = 0; while(i < 10) { if(i == 6) { break; } System.out.println("i: " + i); i++; } System.out.println("Final value of i: " + i); } }
The final value of i is 6.
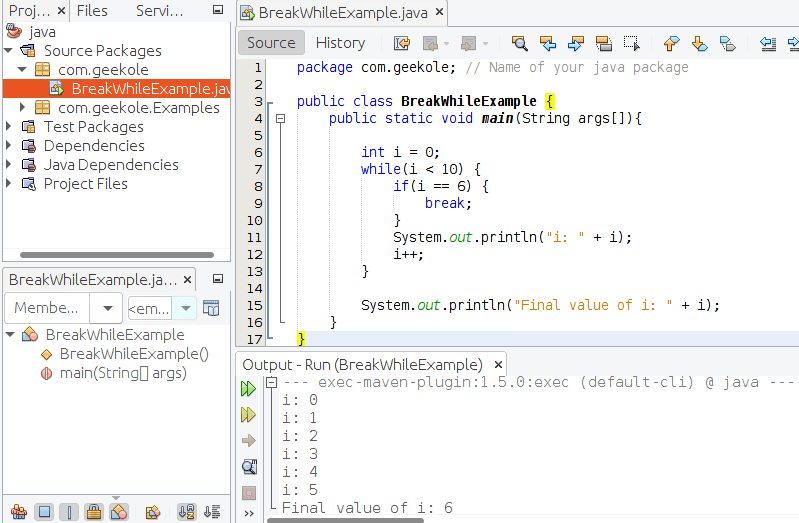
Continue
The continue statement, unlike break, does not interrupt the execution of the loop, but it does interrupt the current iteration. In the following example an iteration does not complete:
package com.geekole; // Name of your java package public class ContinueForExample { public static void main(String args[]){ for (int i = 0; i < 10; i++) { if(i == 6) { continue; } System.out.println("i: " + i); } } }
You will notice that a sequence from 0 to 9 is printed, skipping the printing of 6.
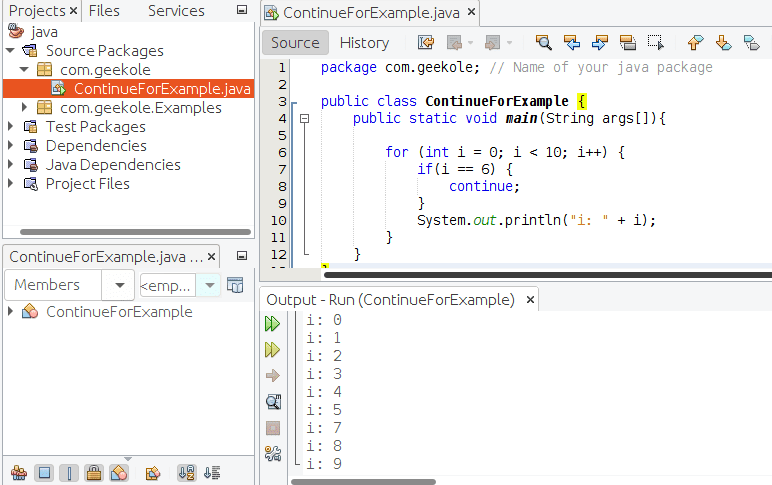
The same thing happens with the while loop, although this time we tried do-while, we show you an example:
package com.geekole; // Name of your java package public class ContinueDoWhileExample { public static void main(String args[]){ int i = 0; do { i++; if(i == 6) { continue; } System.out.println("i: " + i); } while(i < 10); } }
If you run the code:
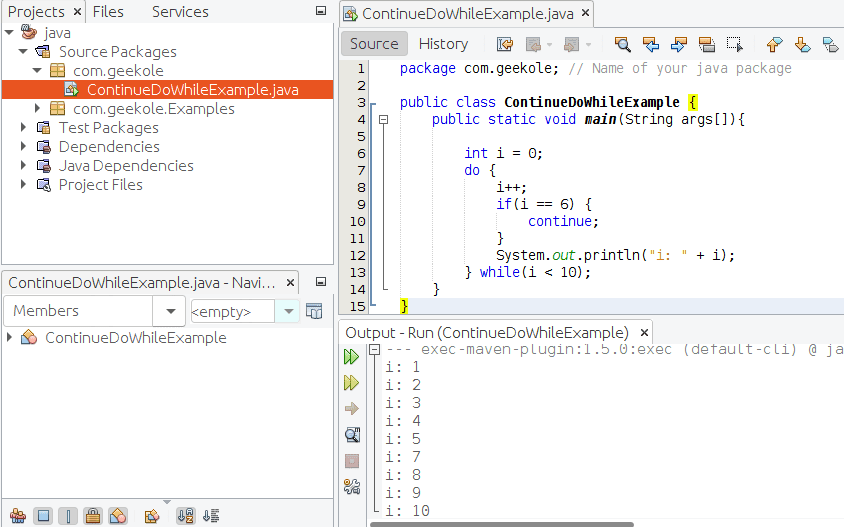
As in the previous example with the for loop, the printout of 6 is skipped.
You must be careful because you can iterate indefinitely if the position in which the continue statement is placed prevents the condition from being met.
In the following example the iterations never stop:
package com.geekole; // Name of your java package public class ContinueWhileExample { public static void main(String args[]){ int i = 0; while (i < 10) { System.out.println("i: " + i); if(i == 2) { continue; } i++; } } }
It will be necessary to stop the virtual machine to stop the execution of the code.
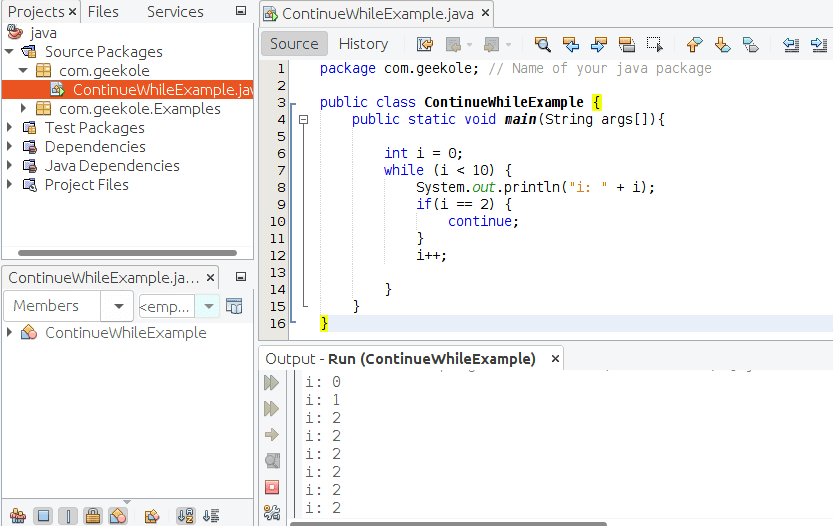
We hope these examples will help you to understand the use of break and continue in Java.