We show you the code to connect to MongoDB in Golang. But before running this example, you need to have a database and a collection to connect. The following exercise was executed with the help of Visual Studio Code and MongoDB Compass
go mod init yourdomain.com/connectmmongodb
Having MongoDB correctly installed, we start the server with the port set by default with the following command in the terminal:
mongod
After we generate a local database in Compass:
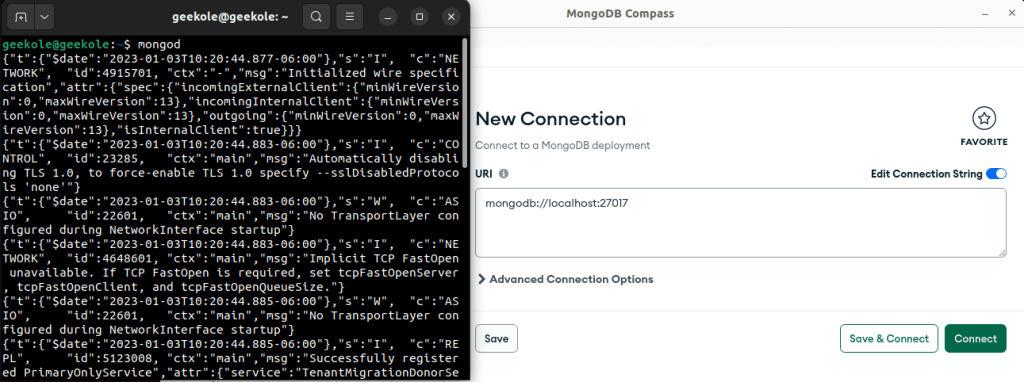
We will also create a database and as required, a new collection.
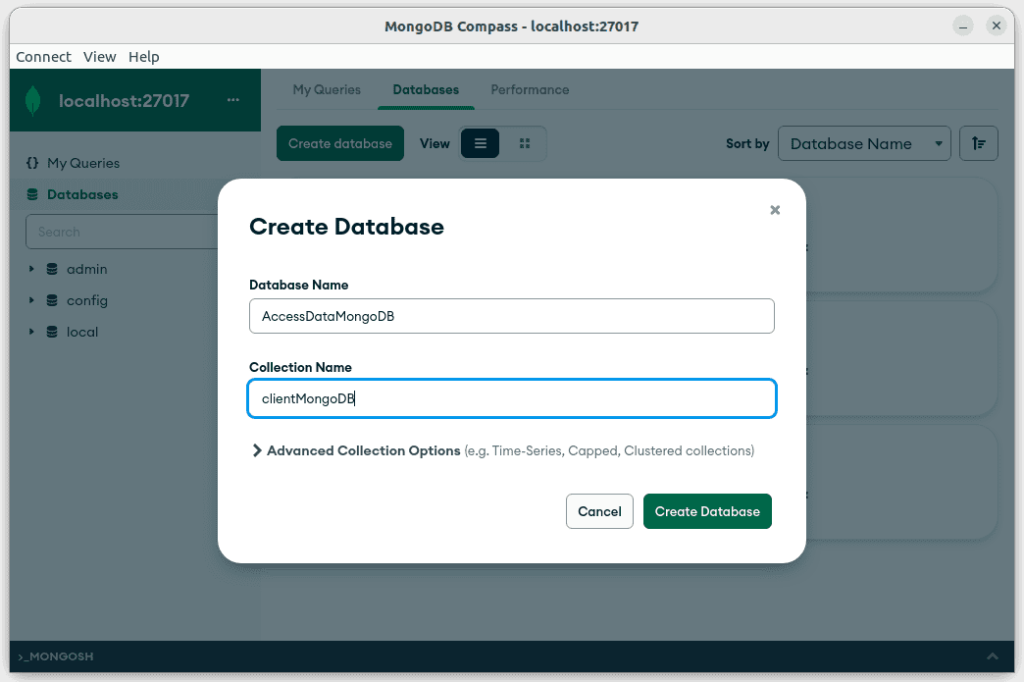
Finally in our collection we insert a new document with test data.
/** * geekole.com */ [{ "name":"Sophia Whatson", "phone":"2255889977", "state":"California" }, { "name":"James Hopkins", "phone":"8899552277", "state":"Texas" }]
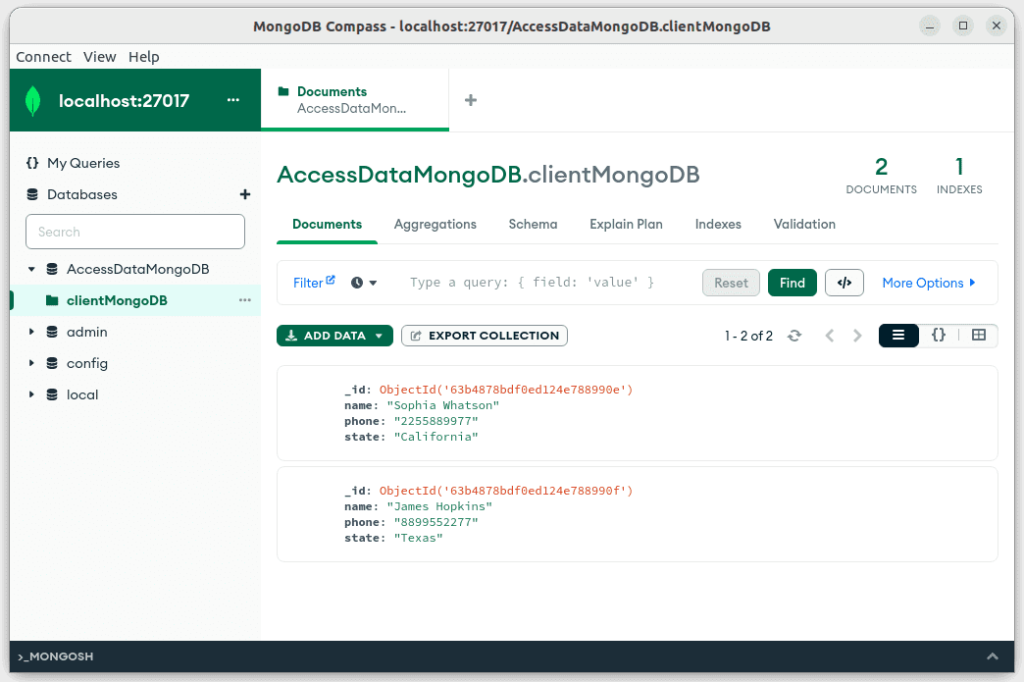
Now the code:
package main import ( "context" "log" "go.mongodb.org/mongo-driver/mongo" "go.mongodb.org/mongo-driver/mongo/options" ) //geekole.com // const AccessDataMongoDB = "mongodb://min01:min01@" + ipServer + ":27017/?authSource=cfdis&readPreference=primary&appname=MongoDB%20Compass&directConnection=true&ssl=false" const AccessDataMongoDB = "mongodb://docker:mongopw@12.12.12.12:49153" var clientMongoDB *mongo.Client func InitMDB() { clientOptions := options.Client().ApplyURI(AccessDataMongoDB) var err error clientMongoDB, err = mongo.Connect(context.TODO(), clientOptions) if err != nil { log.Fatal(err) } //Check the connections err = clientMongoDB.Ping(context.TODO(), nil) if err != nil { log.Fatal(err) } }
To get the libraries execute the following command inside the terminal of your project in Visual Studio Code:
go get go.mongodb.org/mongo-driver/mongo
If you got an error with git, you can try running the following code:
sudo apt-get install git
Having as a final result the following:
We hope this example of how to Connect to MongoDB in Golang will help you.