Here’s an example of how to convert a struct to JSON in Golang using Marshal with the encode/json package. Whether you need it as a string to send or to store, a single line is enough.
Here goes the code:
package main import ( "encoding/json" "fmt" ) //geekole.com type User struct { Name string User string Password string Email string } func main() { user := &User{Name: "Michael", User: "Mich", Password: "123", Email: "test@mail.com"} userJSON, err := json.Marshal(user) if err != nil { fmt.Printf("Error: %s", err) return } fmt.Println(string(userJSON)) }
In this example we have created a struct called User, with typical example fields. It is important that the variables Name, User, Password and Email begin with a uppercase letters. Otherwise they will not be taken into account for the conversion. This is the way we say in Go that a variable can be used outside of the package it was declared in or can be exported.
Another thing you can add is the name it should have for the conversion or the name as a JSON field, we do this by adding a description string after the declaration, we modify the example:
package main import ( "encoding/json" "fmt" ) //geekole.com type User struct { Name string `json:"name"` User string `json:"user"` Password string `json:"password"` Email string `json:"email"` } func main() { user := &User{Name: "Michael", User: "Mich", Password: "123", Email: "test@mail.com"} userJSON, err := json.Marshal(user) if err != nil { fmt.Printf("Error: %s", err) return } fmt.Println(string(userJSON)) }
Single quotes are used to declare that it is a JSON field followed by a colon and between double quotes, the name it will have after the conversion, which can be totally different:
type User struct { Name string `json:"name"` User string `json:"user"` Password string `json:"password"` Email string `json:"email"` }
El resultado en consola será algo como esto:
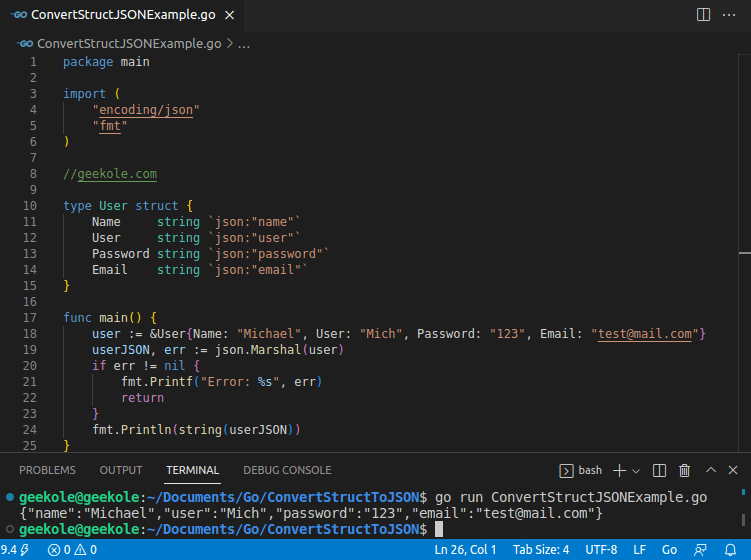
It is not complicated to convert a struct to JSON in Golang, we hope this example will help you.
See more documentation: https://pkg.go.dev/encoding/json@go1.17