In this example we show you how to copy files from one folder to another using Java with the help of InputStream and OutputStream. To show how to copy files we will use a text file called «original.txt» which only contains a few lines.
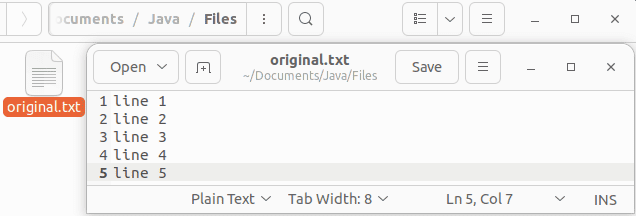
The code to Copy files in Java is the following:
package com.geekole; // Name of your java package import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.io.InputStream; import java.io.OutputStream; /** * * @author geekole.com */ public class CopyFile { public static void main(String[] args) { InputStream inputStream = null; OutputStream outputStream = null; try { File originalFile = new File("/path/original.txt"); File fileCopy = new File("/path/copy.txt"); inputStream = new FileInputStream(originalFile); outputStream = new FileOutputStream(fileCopy); byte[] buffer = new byte[1024]; int length; while ((length = inputStream.read(buffer)) > 0) { outputStream.write(buffer, 0, length); } inputStream.close(); outputStream.close(); System.out.println("File copied."); } catch (IOException e) { e.printStackTrace(); } } }
As you can see, with the help of FileInputStream and its read method, we read a segment of the file in a byte array called a buffer, with the help of a while loop, at the same time we read, we write the content using the write method of FileOutputStream. Last but not least, we close inputStream and outputStream with their close methods.
When we run the code we get the following:
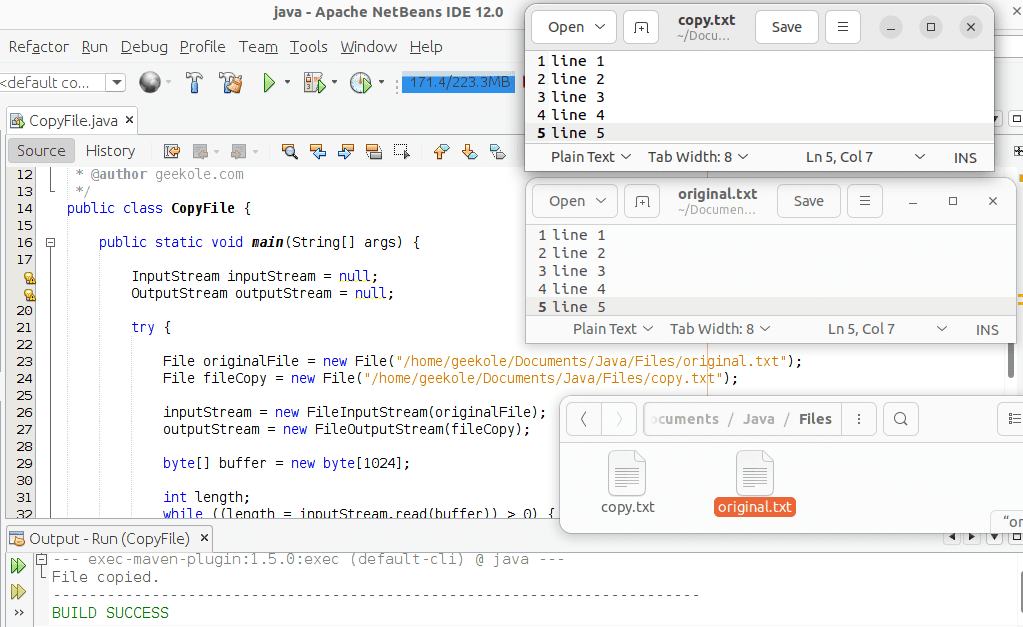
We will have a copy of the file «original.txt» but with the name «copy.txt».
There are other ways to copy files, this is one of the ones that allows you more control over the information by copying byte arrays. We hope you find it useful.