To create a Web Server with Golang does not require a lot of code, although you should be familiar with basic concepts of communication over http. You also need to know that for large applications it is better to use a Framework, so that it facilitates the recognition and control of user requests in a more robust way as Gin does. However, sometimes it is useful to have full control of each request and to start, we show you and explain how to do it.
The code is the following:
package main import ( "fmt" "log" "net/http" ) //geekole.com func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, welcome to %s!", r.URL.Path[1:]) } func main() { http.HandleFunc("/url_test", handler) log.Fatal(http.ListenAndServe(":8080", nil)) }
In main function we call http.HandleFunc, which tells the http package to handle all requests to the web root (“/url_test”) with the “handler”.
http.HandleFunc("/url_test", handler)
Subsequently, http.ListenAndServe is called, specifying that it should listen for requests on port 8080 on any interface (“:8080”). Don’t worry about the second parameter now.
log.Fatal(http.ListenAndServe(":8080", nil))
ListenAndServe always returns an error, but it will be returned when an unexpected error occurs in some request. To log that error, we wrap the function call with log.Fatal. The function handler is of type http.HandlerFunc. It takes an http.ResponseWriter and an http.Request as arguments. Our argument w of type http.ResponseWriter assembles the response from the HTTP server; sending it via HTTP to the client. The argument r of type http.Request is a data structure that represents the HTTP request from the client. The r.URL.Path parameter is the path component of the request URL. The string “[1:]” at the end means “create a Path sub-segment from the first character to the end”. This removes the leading “/” from the path name.
Don’t worry so much if you don’t understand now what’s going on.
If you run the example in the browser with the URL:
http://localhost:8080/url_test
You will get the following response in the browser:
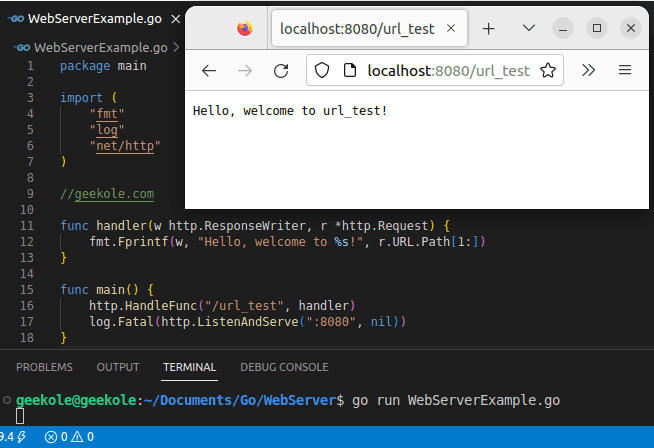
The message it returns is the following:
Hello, welcome to url_test!
You can change the end of the URL and try different ones.
Something important that you must take into account is that you are using a port that is not normally used, 8080. Therefore, it is possible that your operating system requires confirmation for its use if you are in Windows or, you must grant it permissions from the Firewall in the case of Linux. We hope this simple example of how to create a Web Server with Golang will help you to get started.
See more documentation: https://golang.org/doc/articles/wiki/