In this example we are going to create an interface with PySimpleGUI in Python. You can use the PySimpleGUI package to create beautiful interfaces that users will enjoy. PySimpleGUI is a new Python GUI library that has recently gained a lot of interest.
Install PySimpleGUI
To install PySimpleGUI from pip you can use the following command:
pip install pysimplegui
You may need to install tkinter, since PySimpleGUI is a Wrapper for tkinter.
pip install tk
In the first example we will create a window with the title “Hello World“.
import PySimpleGUI as sg #geekole.com sg.Window(title= "Hello World", layout=[[]], margins=(100,80)).read()
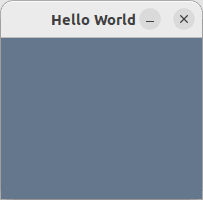
In this second example we will generate a window but now we add a button with the help of a layout, which is simply a container of graphic elements that in this case is a text label and the button with the legend “Ok“.
import PySimpleGUI as sg #geekole.com layout = [ [sg.Text("Hello from PySimpleGUI")], [sg.Button("Ok")] ] window = sg.Window("Demi", layout) while True: event, values = window.read() if event == "Ok" or event == sg.WIN_CLOSED: break window.close()
The result will be the following:
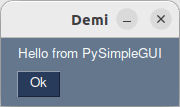
Finally a more complete example, where we will also display images in png or gif format (jpg is not supported with tkinter):
import PySimpleGUI as sg import os.path from PySimpleGUI.PySimpleGUI import FolderBrowse, Listbox #geekole.com file_list_column = [ [ sg.Text("Image Directory"), sg.In(size=(25, 1), enable_events=True, key="-FOLDER-"), sg.FolderBrowse() ], [ sg.Listbox( values=[], enable_events=True, size=(40, 20), key="-FILE LIST-" ) ] ] image_viewer_column = [ [sg.Text("Choose the image you want to view:")], [sg.Text(size=(100,1), key="-TOUT-")], [sg.Image(key="-IMAGE-")] ] layout = [ [ sg.Column(file_list_column), sg.VSeparator(), sg.Column(image_viewer_column) ] ] window = sg.Window("Visualizer", layout) while True: event, values = window.read() if event == "Exit" or event == sg.WIN_CLOSED: break if event == "-FOLDER-": folder = values["-FOLDER-"] try: file_list = os.listdir(folder) except: file_list =[] fnames = [ f for f in file_list if os.path.isfile(os.path.join(folder, f)) and f.lower().endswith((".png",".gif")) ] window["-FILE LIST-"].update(fnames) elif event == "-FILE LIST-": try: filename = os.path.join( values["-FOLDER-"], values["-FILE LIST-"][0] ) window["-TOUT-"].update(filename) window["-IMAGE-"].update(filename=filename) except: pass window.close()
In this last example we add several elements, among which is a FolderBrowse and a Listbox in a first column. On the right side we have an Image element, where our selected image will appear.
When selecting a folder with valid images, the list with the files that we can view will appear.
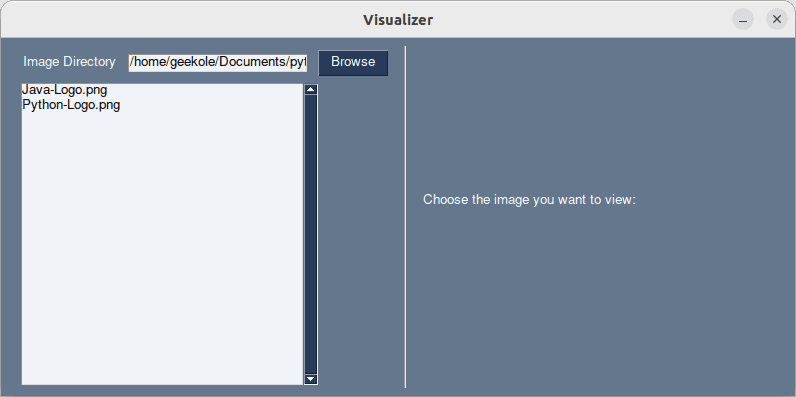
By clicking on the item in the list you can view the image:
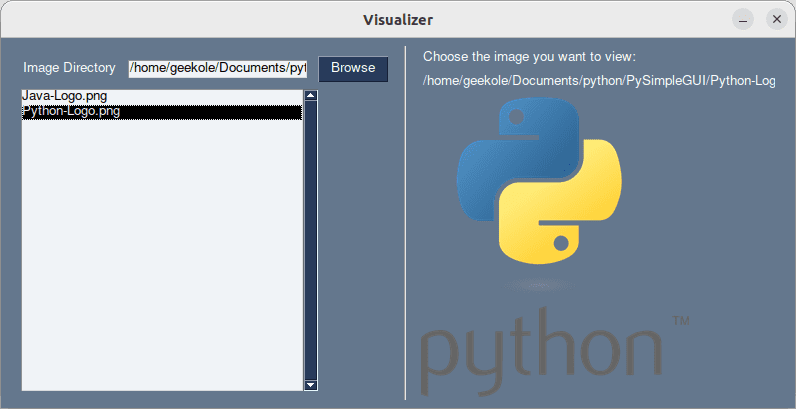
We hope this examples of how to Create an interface with PySimpleGUI will be useful for you, soon we will make more examples of this library.
See more documentation: https://pysimplegui.readthedocs.io/en/latest/
See more examples: https://github.com/PySimpleGUI/PySimpleGUI/tree/master/DemoPrograms