It is possible to create an XML file in Golang from a struct, we will show you how to do it. Of course it is possible to create an XML simply by generating a string with the nodes you require and then writing it to a file, but with the following method you will be sure that your node structure will be well formed and you will use one of the data handling features Golang’s most powerful.
You can copy and try the following code:
package main import ( "encoding/xml" "fmt" "log" "os" ) //geekole.com type User struct { XMLName xml.Name `xml:"user"` Id int `xml:"id,attr"` Name string `xml:"name"` Username string `xml:"username"` Password string `xml:"password"` Email string `xml:"email"` } func main() { user := &User{Id: 1, Name: "Jhon", Username: "jhon", Password: "123", Email: "test@mail.com"} out, _ := xml.MarshalIndent(user, " ", " ") fmt.Println(string(out)) f, err := os.Create("/path/file.xml") if err != nil { log.Fatal(err) } defer f.Close() _, err2 := f.WriteString(string(out)) if err2 != nil { log.Fatal(err2) } fmt.Println("Ready") }
In the first block of code we define the structure that we want to convert to XML nodes:
type User struct { XMLName xml.Name `xml:"user"` Id int `xml:"id,attr"` Name string `xml:"name"` Username string `xml:"username"` Password string `xml:"password"` Email string `xml:"email"` }
Our User struct has several elements. If these elements will be attributes in a tag, they must be indicated as in the case of the Id, after the data type xml:”id,attr”, with the string “attr” after the comma.
The other nodes that contain information must indicate the name that the delimiter tags will have, as in the case of Name: xml:”username”.
Later we use the MarshalIndent from the encoding/xml package.
out, _ := xml.MarshalIndent(user, " ", " ")
In the following lines we create, write and close our text file with the extension “.xml“
f, err := os.Create("/home/geekole/Documents/go/files/file.xml") if err != nil { log.Fatal(err) } defer f.Close() _, err2 := f.WriteString(string(out)) if err2 != nil { log.Fatal(err2) } fmt.Println("Ready")
Of course, you must change the path where you want the file to be generated. Running the example will generate an XML file like the one shown below:
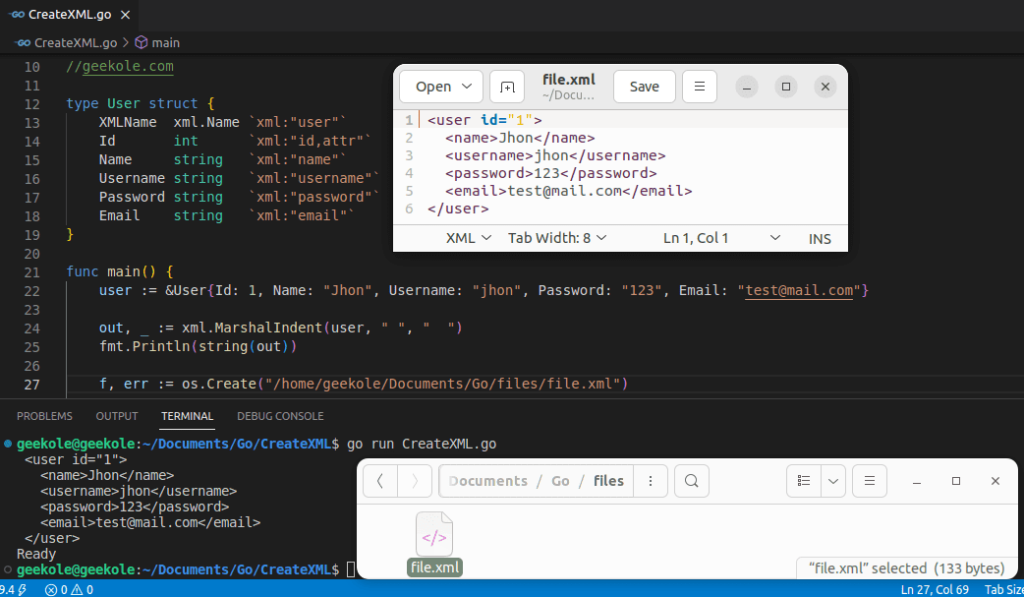
This example to create an XML file in Golang is simple, of course the final file can be as complex as you want, as long as you correctly define the sctucts that you need to convert to XML.
See more: https://golang.org/doc/