In Java a folder or directory can be created with the help of mkdir method and it allows you to create one or more directories recursively. Let’s see an example for the first case:
package com.geekole; // Name of your java package import java.io.File; /** * * @author geekole.com */ public class ExampleCreateDirectory { public static void main(String args[]){ File directory = new File("/path/new_directory"); if (!directory.exists()) { if (directory.mkdirs()) { System.out.println("Directory created"); } else { System.out.println("Error creating directory"); } } } }
In this example we create a directory called new_directory, the path will obviously need to be changed in your example.
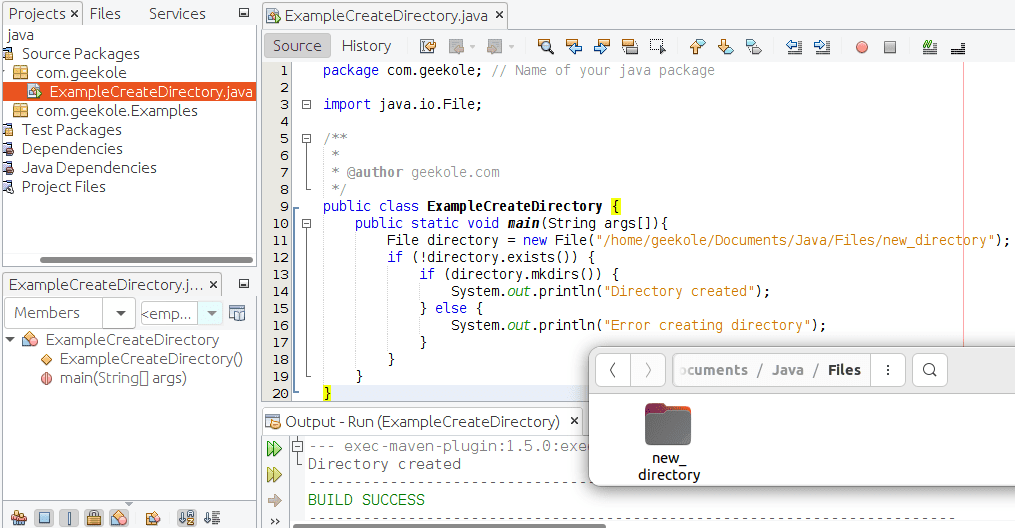
Create multiple folders
The mkdir method also allows you to create multiple directories when they are successively inside one another.
package com.geekole; // Name of your java package import java.io.File; /** * * @author geekole.com */ public class ExampleCreateDirectories { public static void main(String args[]){ File directories = new File("/path/dir1/dir2"); if (!directories.exists()) { if (directories.mkdirs()) { System.out.println("Multiple directories were created"); } else { System.out.println("Error creating directories"); } } } }
As you can see, two directories have been created in the same statement: dir1 and dir2.
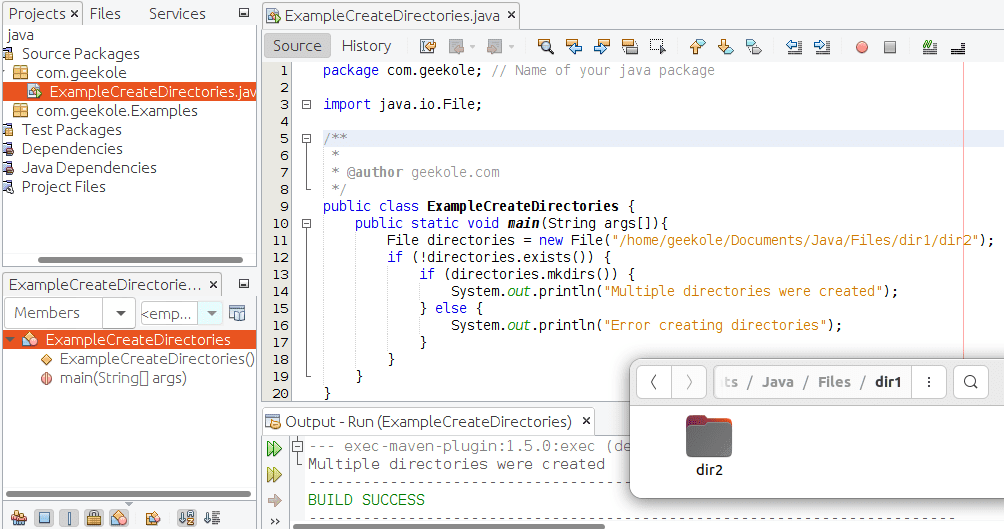
Of course, these operations depend on the read and write permissions you have, so you should consider it when writing your code. On the other hand, in both examples we always check for the existence of the folder before trying to create it with the help of the exists method.
Note: the examples to Create directory or folder in Java are edited and executed in NetBeans.