Using cElementTree it is possible to create an XML file in Python. In the following example we create the root node with the Element(“root”) function.
We then create a new element inside root called «doc» with the SubElement function.
import xml.etree.cElementTree as ET root = ET.Element("root") doc = ET.SubElement(root, "doc") node1 = ET.SubElement(doc, "node1", name="node") node1.text = "Node 1 text" ET.SubElement(doc, "node2", attribute="something").text = "text 2" tree = ET.ElementTree(root) tree.write("/path/test.xml")
To add new nodes we again use SubElement with which we get a reference to node1 on line 6 and assign a text string “Text of node1”.
Once we finish adding nodes to our XML document, we call ElementTree, which returns a reference to the complete document, and we create the text file with our XML using the write function, which receives as an argument the path where the XML file will be created.
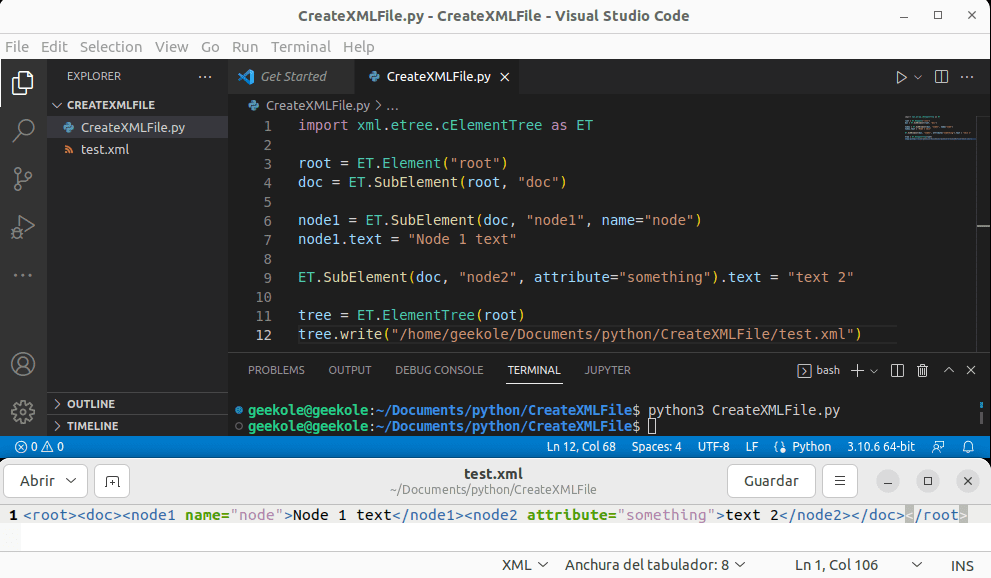
The result will be the following:
<root><doc><node1 name="node">Node 1 text</node1><node2 attribute="something">text 2</node2></doc></root>
We hope this example of how to create an XML file in Python will help you.