To create a ZIP files in Java we do not need to import additional libraries in Maven, the util package provides the necessary classes to create a ZIP file.
import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.util.zip.ZipEntry; import java.util.zip.ZipOutputStream;
We will use any file to compress.
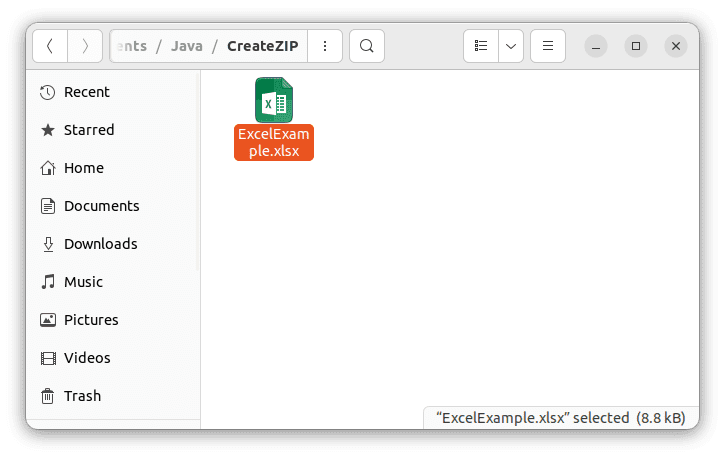
The code to create ZIP files in java:
package com.geekole; // Name of your java package import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.util.zip.ZipEntry; import java.util.zip.ZipOutputStream; /** * * @author geekole.com */ public class CreateZIP { public static void main( String[] args ) { byte[] buffer = new byte[1024]; try{ FileOutputStream fos = new FileOutputStream("/files_path/zip_file.zip"); ZipOutputStream zos = new ZipOutputStream(fos); ZipEntry ze= new ZipEntry("ExcelExample.xlsx"); zos.putNextEntry(ze); FileInputStream in = new FileInputStream("/files_path/ExcelExample.xlsx"); int len; while ((len = in.read(buffer)) > 0) { zos.write(buffer, 0, len); } in.close(); zos.closeEntry(); zos.close(); System.out.println("Done"); }catch(IOException ex){ ex.printStackTrace(); } } }
The lines you should pay special attention to are the following:
The FileOutputStream class is used to create the ZIP file with our files, which in this case is just one.
FileOutputStream fos = new FileOutputStream("/files_path/zip_file.zip");
The ZipOutputStream class allows you to compress each of the entries generated from the ZipEntry class with the name of the original file or whatever you want. Of course we have to create a FileInputStream where we specify the path of the file we want to compress.
ZipOutputStream zos = new ZipOutputStream(fos); ZipEntry ze= new ZipEntry("ExcelExample.xlsx"); zos.putNextEntry(ze); FileInputStream in = new FileInputStream("/files_path/ExcelExample.xlsx");
We will write the content of our source file with the write and ZipOutputStream function takes care of doing the compression.
int len; while ((len = in.read(buffer)) > 0) { zos.write(buffer, 0, len); } in.close(); zos.closeEntry(); zos.close();
Don’t forget to close the streams and the input.
If we run the example we get the following:
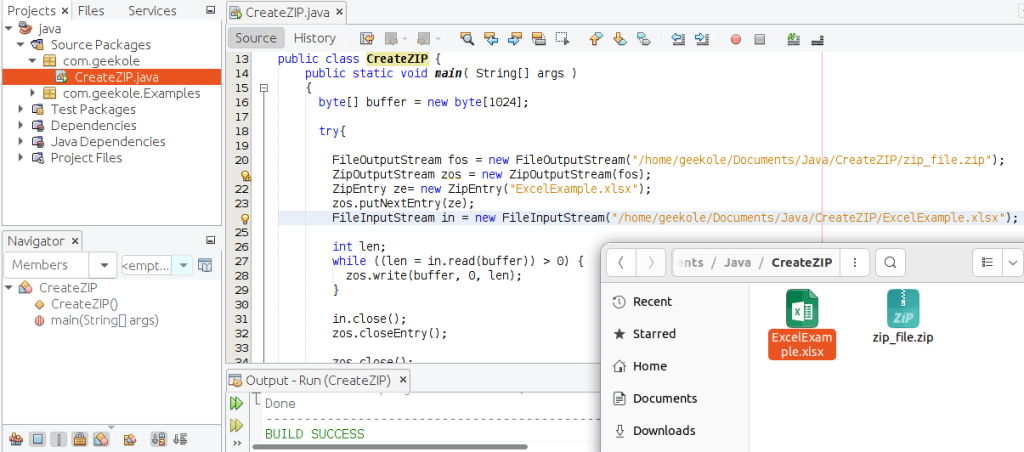
The file zip_file.zip will contain the example file and of course you can use any other.