In this example we will see how to create a ZIP files in Python, we will compress four text files, but the example can be done with any type and amount of files or files:
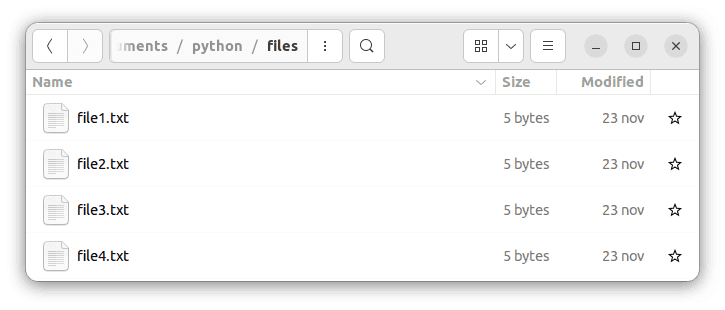
In our code we import the zipfile package.
import zipfile # geekole.com try: import zlib compression = zipfile.ZIP_DEFLATED except: compression = zipfile.ZIP_STORED zf = zipfile.ZipFile("/home/geekole/Documents/python/files/example_file.zip", mode="w") try: zf.write("/home/geekole/Documents/python/files/file1.txt", compress_type=compression) zf.write("/home/geekole/Documents/python/files/file2.txt", compress_type=compression) zf.write("/home/geekole/Documents/python/files/file3.txt", compress_type=compression) zf.write("/home/geekole/Documents/python/files/file4.txt", compress_type=compression) finally: zf.close()
The lines of code that you must modify are the following:
You must first specify that the archive will be compressed, by importing the zlib module and defining the storage type in the ZIP, using
compression = zipfile.ZIP_DEFLATED
You must do this within a try except statement as this step may generate an error in which case the compression type will change.
try: import zlib compression = zipfile.ZIP_DEFLATED except: compression = zipfile.ZIP_STORED
We then create the example ZIP file.
zf = zipfile.ZipFile("/home/geekole/Documents/python/files/example_file.zip", mode="w")
To each file we add it using the write function.
zf.write("/home/geekole/Documents/python/files/file1.txt", compress_type=compression) zf.write("/home/geekole/Documents/python/files/file2.txt", compress_type=compression) zf.write("/home/geekole/Documents/python/files/file3.txt", compress_type=compression) zf.write("/home/geekole/Documents/python/files/file4.txt", compress_type=compression)
The result will be something like this:
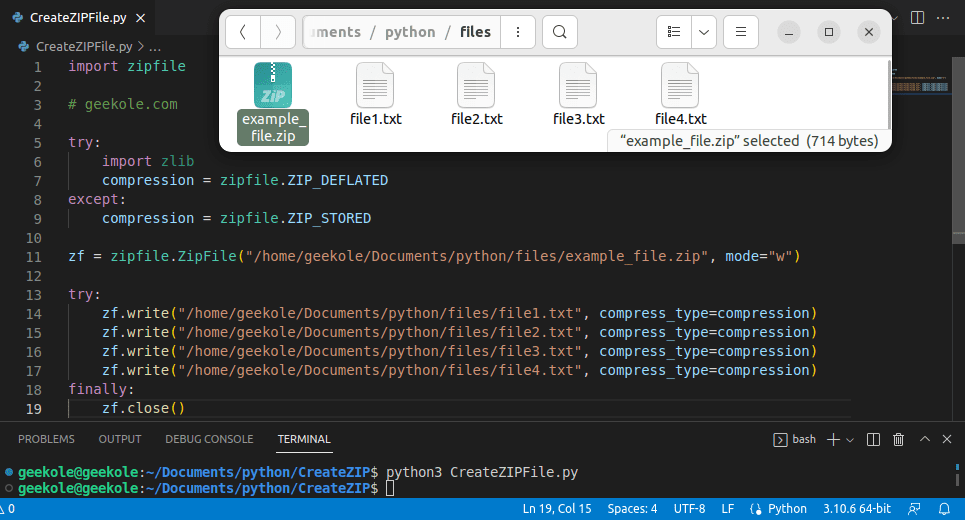
The file example_file.zip will contain the files that we specify in code: file1.txt, file2.txt, file3.txt and file4.txt.
And that’s it, this is a simple example about create ZIP files in python, which we hope will be useful to you.