To delete a file in java we use the delete method of the File class. Having two possible options shown in the following code:
package com.geekole; // Name of your java package import java.io.File; /** * @author geekole.com */ public class ExampleDeleteFile { public static void main(String args[]){ File file1 = new File("/path/filename1.txt"); file1.delete(); File file2 = new File("/path/filename2.txt"); file2.deleteOnExit(); } }
Actually in the code that we show you we have two ways to delete a file or file. In the first example where we create the File file1 instance that references filename1.txt , we delete immediately after creating the instance using delete and in the second example we do the same thing but use the deleteOnExit method to delete the file filename2.txt.
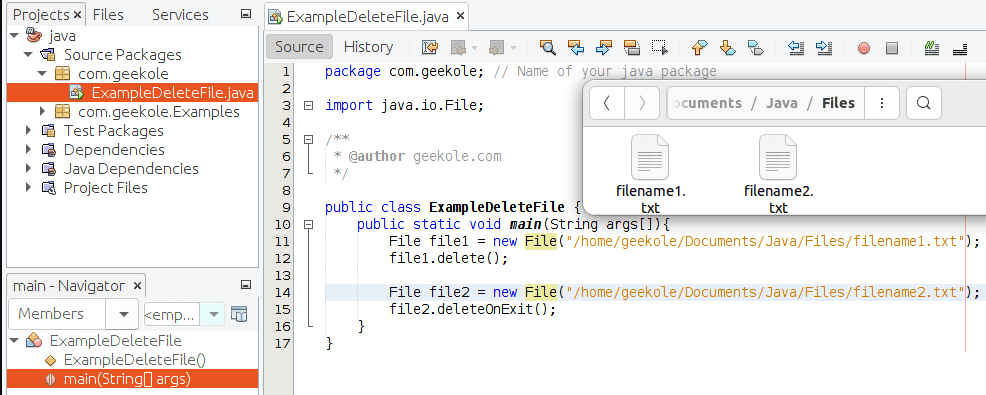
When we run the code, the files will be deleted.
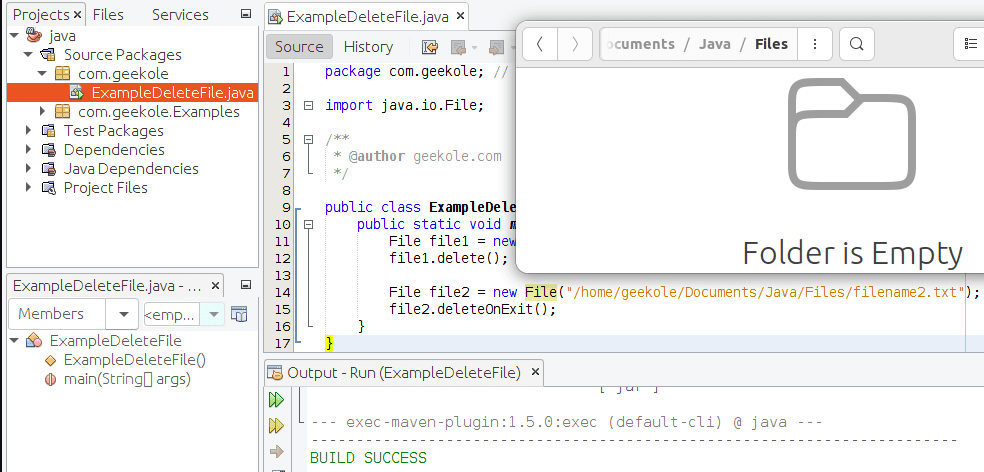
The Java documentation says the following about it:
Delete method
Deletes the file or directory indicated by the path. If this path denotes a directory, then the directory must be empty to be removed.
Note that the java.io.file.Files class defines the delete method to throw an IOException when a file cannot be deleted. This is useful for reporting errors and diagnosing why a file cannot be deleted.
DeleteOnExit method
Requests that the file or directory indicated by this abstract path be removed when the virtual machine terminates. Files (or directories) are removed in the reverse order in which they are registered. Invoking this method to delete a file or directory that is already checked in for deletion has no effect. The removal will be attempted only during normal termination of the virtual machine, as defined in the Java language specification.
However, when deleting files there are some points that you should consider, the file may not be deleted if it is being used and in some operating systems an error will not even be generated.