Error and Exception handling in Python is one of the most important topics. A typical exception handling code block will have the following form:
try: #Code with possible Exception except ValueError: #Exception handling
Perhaps some of the errors that you will most frequently find are syntax errors, also known as interpretation errors, and they are perhaps the most common type of error when you are learning the language. If you execute the following lines of code in the interpreter you will get a SintaxError: invalid syntax:
>>> i = 0 >>> if i < 5 print('i is less than 5') File "<stdin>", line 1 if i < 5 print('i is less than 5') ^ SyntaxError: invalid syntax >>>
And it is because it has simply been necessary to put a colon after the If. Errors like this are typical and it is very useful that Python alerts you and indicates the position where they have occurred with a small arrow.
However, not all errors that exist will be syntax errors, there are also Exceptions.
Exceptions
It is very likely that even if everything is written correctly, Exceptions may arise during the execution of our program, since many times the input data varies and its value may not be what is expected. Let’s look at some examples:
>>> 100 * (200/0) Traceback (most recent call last): File "<stdin>", line 1, in <module> ZeroDivisionError: division by zero
We have gotten a ZeroDivisionError for a division by zero.
>>> 5 + undefined_variable * 2 Traceback (most recent call last): File "<stdin>", line 1, in <module> NameError: name 'undefined_variable' is not defined
We get a NameError for a variable not previously defined.
>>> 'total_amount' + 10 Traceback (most recent call last): File "<stdin>", line 1, in <module> TypeError: can only concatenate str (not "int") to str
A TypeError due to mishandling of the “+” operator when trying to concatenate strings with an integer.
Exceptions can stop your program from running, but they don’t have to, we’ll see how to handle them.
In the following example we see the most elaborate typical structure of an Exception Handling Block.
while True: try: x = int(input("Enter a number: ")) break except ValueError: print("It is not a valid number, please try again.")
On this block, you can see a try statement, which is how we indicate that we are going to handle a potential exception.
In case the code contained in the try block throws an exception, it will be handled by the code in «except».
If no exception occurs, execution of our except block is skipped.
If an Exception occurs that is not included in our except statement, we will have an unhandled exception, which can stop the execution of the program, you can use more than one except.
Running the example in the interpreter we will obtain the following behavior:
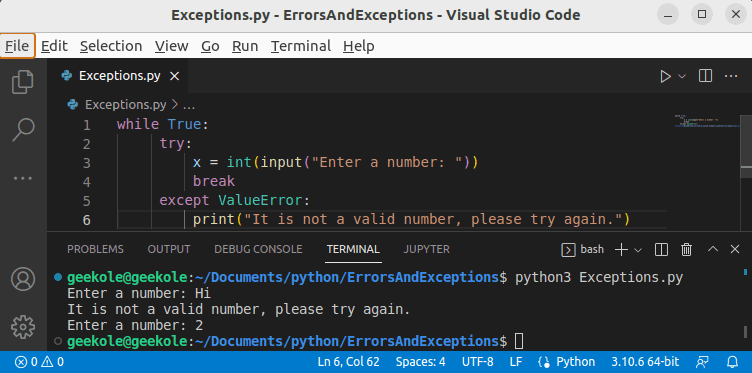
As mentioned above, it is possible to handle more than one exception by adding as many except statements as necessary.
import sys try: f = open('file.txt') s = f.readline() i = int(s.strip()) except OSError as err: print("OS error: {0}".format(err)) except ValueError: print("It is not possible to convert this string to integer") except: print("Unexpected error:", sys.exc_info()[0]) raise
In the previous example, we see that we can also use the except statement as a wildcard, when we do not know the error or exception specifically.
Throw your own Exceptions
You may want to throw your own exceptions, to do so you can use the raise statement. For example:
>>> raise NameError('Unexpected error') Traceback (most recent call last): File "<stdin>", line 1, in <module> NameError: HiThere
You can create your own Exceptions, these are classes that inherit from Exception and you simply have to call raise as follows:
raise OperacionError #Assuming you declare it elsewhere
Finally, the handling of Errors and Exceptions in Python is essential and you should apply it whenever you consider it important to control the execution and flow of your program so that it does not stop unexpectedly.
Más información en la pagina oficial: https://docs.python.org/3/tutorial/errors.html