To read or extract files from a ZIP in Java we do not need to import additional libraries in Maven, the util package provides the necessary classes to extract information from a ZIP file.
import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.util.List; import java.util.zip.ZipEntry; import java.util.zip.ZipInputStream;
We will use this ZIP file with four text files, but in principle you can use any ZIP file.
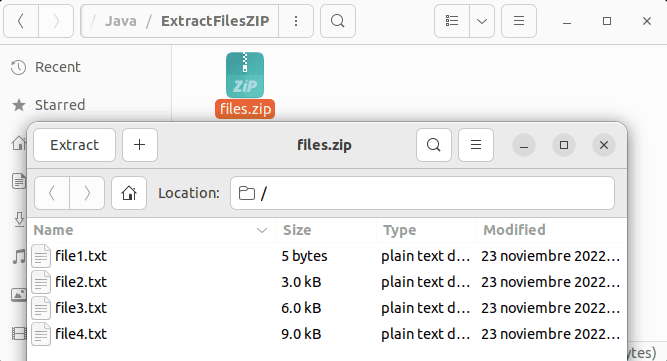
The code to extract files from a ZIP in Java:
package com.geekole; // Name of your java package import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.util.List; import java.util.zip.ZipEntry; import java.util.zip.ZipInputStream; /** * * @author geekole.com */ public class ReadZip { List fileList; private static final String FILE_ZIP = "/path/files.zip"; private static final String EXIT_PATH = "/path/"; public static void main(String[] args) { ReadZip readZip = new ReadZip(); readZip.unZip(FILE_ZIP, EXIT_PATH); } public void unZip(String fileZip, String exitPath) { byte[] buffer = new byte[1024]; try { File folder = new File(EXIT_PATH); if (!folder.exists()) { folder.mkdir(); } ZipInputStream zis = new ZipInputStream(new FileInputStream(fileZip)); ZipEntry ze = zis.getNextEntry(); while (ze != null) { String fileName = ze.getName(); File newFile = new File(exitPath + File.separator + fileName); System.out.println("unzipped file : " + newFile.getAbsoluteFile()); new File(newFile.getParent()).mkdirs(); FileOutputStream fos = new FileOutputStream(newFile); int len; while ((len = zis.read(buffer)) > 0) { fos.write(buffer, 0, len); } fos.close(); ze = zis.getNextEntry(); } zis.closeEntry(); zis.close(); System.out.println("Ready"); } catch (IOException ex) { ex.printStackTrace(); } } }
The lines you should pay special attention to are the following:
The paths of the ZIP file from which you are going to extract information and the path where the files extracted from the ZIP will be created.
private static final String FILE_ZIP = "/home/geekole/Documents/java/files/files.zip"; private static final String EXIT_PATH = "/home/geekole/Documents/java/files/";
The files in the ZIP can be browsed using the getNextEntry method.
ZipEntry ze = zis.getNextEntry();
In a while loop you extract the name of the file to later create the file with the same name:
String fileName = ze.getName();
Then create the uncompressed file with a FileOutputStream.
new File(newFile.getParent()).mkdirs(); FileOutputStream fos = new FileOutputStream(newFile); int len; while ((len = zis.read(buffer)) > 0) { fos.write(buffer, 0, len); } fos.close();
If we run the example we get this result:
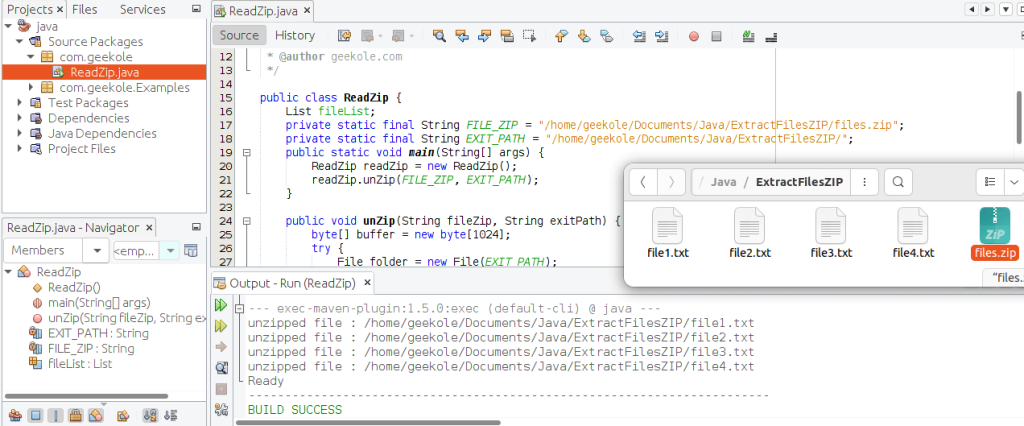
As a result you can see that the four text files contained in our ZIP archive are on the path that we specified in the code.
The example is very simple, but it will easily get you started with extract ZIP files in java.