Here’s an example of extract files from a ZIP in Python and having some text files like the image below:
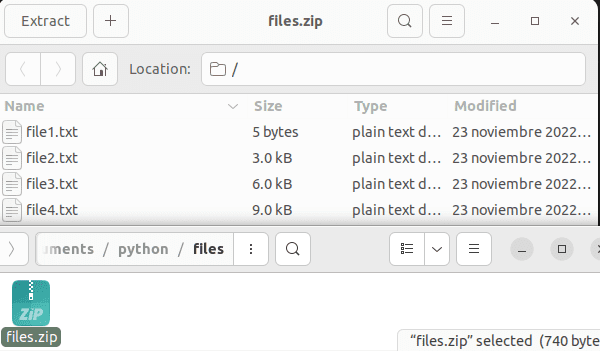
However, you can use the file with whatever content you like.
To start, in our Python code we will import the zipfile package with which we can use “ZipFile”.
import zipfile # geekole.com zip_path = "/home/geekole/Documents/python/files/files.zip" extraction_path = "/home/geekole/Documents/python/files/" password = None zip_file = zipfile.ZipFile(zip_path, "r") try: print(zip_file.namelist()) zip_file.extractall(pwd=password, path=extraction_path) except: pass zip_file.close()
The lines of code that you must modify are the path of the zip file zip_path and the path where the files will be extracted extraction_path and you must replace their values with the paths to files on your own computer. For the extraction path you must make sure you have write permissions.
zip_path = "/home/geekole/Documents/python/files/files.zip" extraction_path = "/home/geekole/Documents/python/files/"
The reference to the ZIP file is constructed with the following statement:
zip_file = zipfile.ZipFile(zip_path, "r")
With the namelist() function, we get the list of files that the ZIP contains.
The extractall() function will allow us to obtain each file contained in the ZIP and receives as arguments a variable with the password, which in our case does not apply, and the file extraction path.
The result will be something like this:
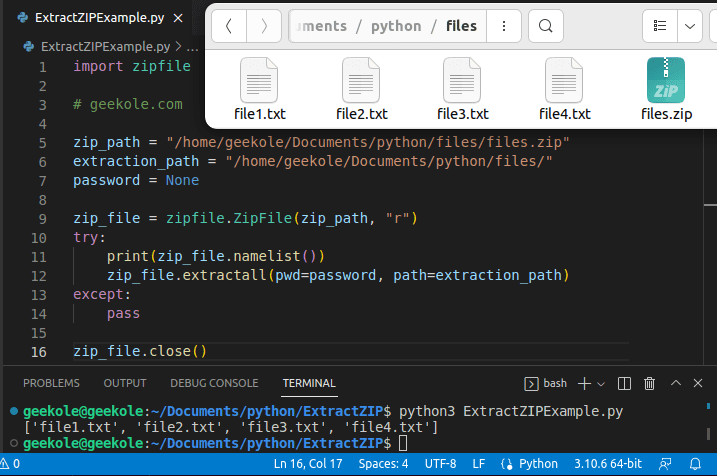
The list of files compressed in the ZIP, but as separate files.
We hope this example of extract files from a ZIP in Python will help you. Not a lot of code is required with Python, in most cases this example will work for you.