For Face Detection with OpenCV in Python for Windows, Debian and Raspberry you can follow these simple steps:
Create a virtual environment in Python
In Linux Debian we use the following command to install virtualenv, which will allow us to create virtual environments and isolate the libraries that we will install so that they do not cause problems:
sudo apt -y install virtualenv
To create a virtual environment we execute the following command:
python3 -m venv /path/to/virtual/environment # Or you can also use this other one if you want to create it in the same folder: python3 -m venv venv
More to how to create an environment or virtual environment in Python: https://decodigo.com/create-a-virtual-environment-in-python
Activate the virtual environment
To activate the virtual environment:
source venv/bin/activate
Install OpenCV
Install OpenCV using pip3:
pip3 install opencv-python
Python code for Windows and Linux
This example was tested on Windows 10 and Debian 11
import cv2 #geekole.com # https://github.com/opencv/opencv/tree/master/data/haarcascades # read the file cascade face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml') # Capture the video cap = cv2.VideoCapture(0) while True: # Read the frame _, img = cap.read() # Converts to grayscale gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # Detecting faces faces = face_cascade.detectMultiScale(gray, 1.1, 4) # Drawing a rectangle for each detected face for (x, y, w, h) in faces: cv2.rectangle(img, (x, y), (x+w, y+h), (255, 0, 0), 2) # Shown in the window cv2.imshow('img', img) # For if the 'Esc' key is pressed k = cv2.waitKey(30) & 0xff if k==27: break # The capture object is released cap.release()
Code for Raspberry with Raspbian
Code for Raspberry with Raspbian modifying the resolution of the capture video, you should also know that other Web cameras can be used. You can use the Camera Pi, however from the latest version of Raspbian (bullseye) the camera will no longer be supported. But for this example you can use any other connected by the USB port.
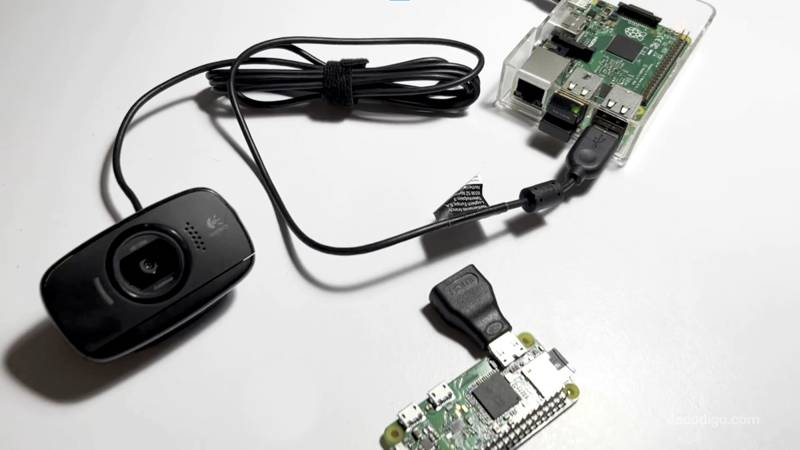
import cv2 #geekole.com # https://github.com/opencv/opencv/tree/master/data/haarcascades # read the file cascade face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml') # Capture the video cap = cv2.VideoCapture(0) cap.set(cv2.CAP_PROP_FRAME_WIDTH, 320) # set new dimensionns to cam object (not cap) cap.set(cv2.CAP_PROP_FRAME_HEIGHT, 240) while True: # Lee el frame _, img = cap.read() # Converts to grayscale gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # Detecting faces faces = face_cascade.detectMultiScale(gray, 1.1, 4) # Drawing a rectangle for each detected face for (x, y, w, h) in faces: cv2.rectangle(img, (x, y), (x+w, y+h), (255, 0, 0), 2) # Shown in the window cv2.imshow('img', img) # For if the 'Esc' key is pressed k = cv2.waitKey(30) & 0xff if k==27: break # The capture object is released cap.release()
Additional
It could happen that you need the following libraries, but if you have no problems it is not necessary to install them
sudo apt-get libatlas-base-dev sudo apt-get install libcblas-dev sudo apt-get install libhdf5-dev sudo apt-get install libhdf5-serial-dev sudo apt-get install libatlas-base-dev sudo apt-get install libjasper-dev sudo apt-get install libqtgui4 sudo apt-get install libqt4-test
As you can see face detection with OpenCV in Python is easy but you can do much more with OpenCV.
More about OpenCV: https://opencv.org/