Doing face mapping with Dlib and Python is quite easy. In addition to being able to detect faces in an image or video, it is sometimes necessary to obtain more detail about the position of the eyes, nose or mouth. You can easily do it with the Dlib library.
Dlib is a facial landmark detector with pre-trained models, Dlib is used to estimate the location of 68 (x,y) coordinates that map facial points on a person’s face like the image below. This example uses a file with the pre-trained model “shape_predictor_68_face_landmarks.dat” which can be saved in the same folder as the example script.
This example was tested on linux debian and additionally I had to install the cmake library.
sudo pip3 install cmake
You must make sure to import the following libraries:
sudo pip3 install numpy opencv-python dlib imutils
And now the code:
from imutils import face_utils import dlib import cv2 # The face detector (HOG) is initialized first, and then # landmarks are added on the detected face # p = It is the reference to the pretrained model, in this case in # the same folder as this script p = "shape_predictor_68_face_landmarks.dat" detector = dlib.get_frontal_face_detector() predictor = dlib.shape_predictor(p) cap = cv2.VideoCapture(0) while True: # The webcam image is obtained _, image = cap.read() # Converting the image to grayscale gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) # The faces of the image are obtained in the webcam rects = detector(gray, 0) # For each face detected, the reference points are searched for (i, rect) in enumerate(rects): # The prediction is made and transformed into a numpy array shape = predictor(gray, rect) shape = face_utils.shape_to_np(shape) # It is drawn on our image, all the coordinates found (x,y) for (x, y) in shape: cv2.circle(image, (x, y), 2, (0, 255, 0), -1) # A window with the image is displayed cv2.imshow("Mapeo de Rostros", image) # The window closes with the key Esc k = cv2.waitKey(5) & 0xFF if k == 27: break cv2.destroyAllWindows() cap.release()
You can download the file: shape_predictor_68_face_landmarks.dat
If you run the script mapping with Dlib and Python you will see several dots on your face:
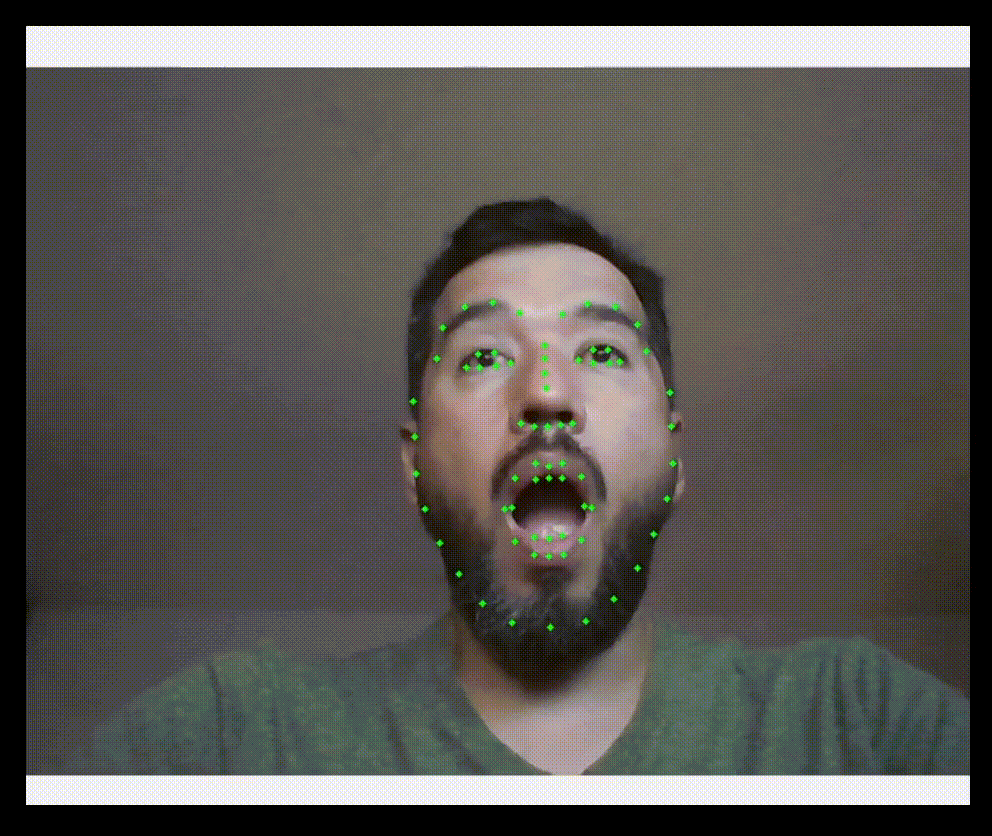
More detail about the file: https://ibug.doc.ic.ac.uk/resources/facial-point-annotations/
More Python examples: https://geekole.com/python/