In Python, the for loop and range() is different from languages like C or Java. Here we show you some examples:
# geekole.com for i in range(5, 10): print(i)
When executing the code we get this result:
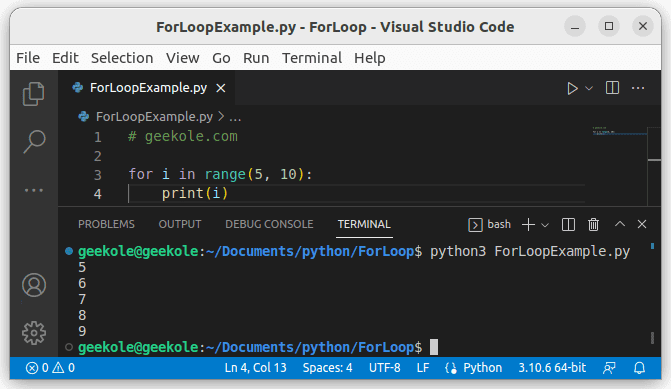
Using the range() function, iterates over a sequence from 5 to 9 (10 is not included).
One way to simplify the use of range() is to use only the maximum value:
# geekole.com for i in range(10): print(i)
The range() function returns values ranging from 0 to 10, it was not necessary to specify that it starts from 0.
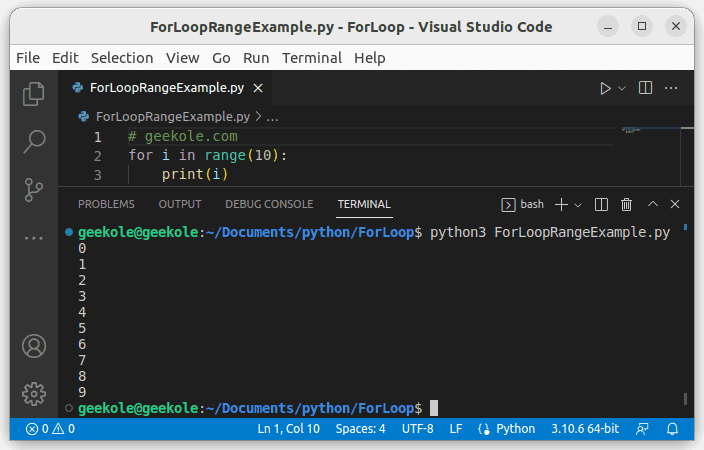
The for loop with the range() function, also allows you to iterate over a range not only by incrementing by 1, you can also increment by specifying a value as shown below:
# geekole.com for i in range(0, 10, 2): print(i) print() for i in range(0, -10, -2): print(i)
The result is two sequences of values where at each iteration the increment is 2 and -2.
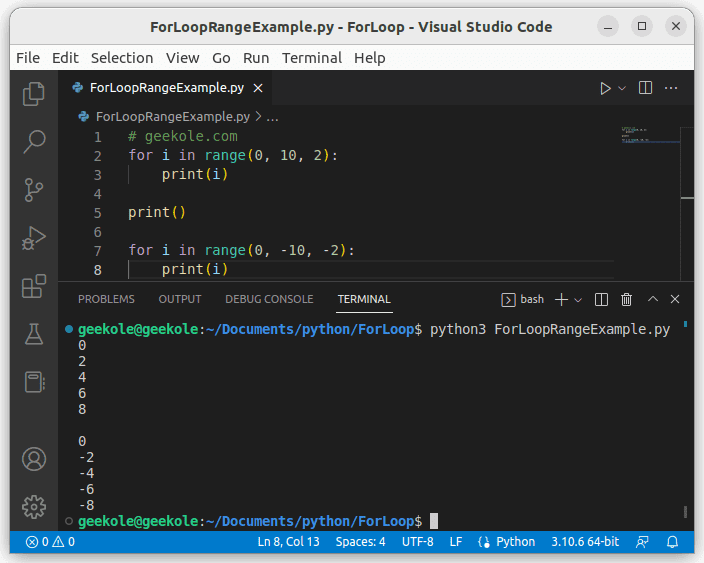
Iterate over a list in Python
Another form of the for loop in Python is the one that allows you to iterate over the elements of a tuple or list.
# geekole.com for i in (3, 5, 3, 6, 100): print(i)
This would be the result:
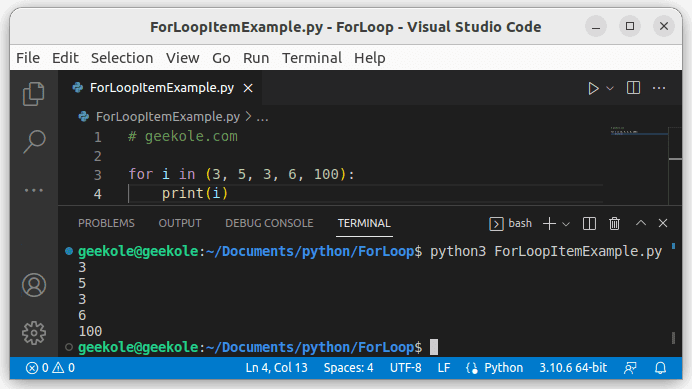
One more variant of for in Python that can be useful depending on the case is one that allows you to execute a block of code once iterations over a list have finished, the for-else loop.
# geekole.com for i in (3, 5, 3, 6, 100): print(i) else: print("Finished")
At the end of the iterations of the for loop, “Finished” will be printed.
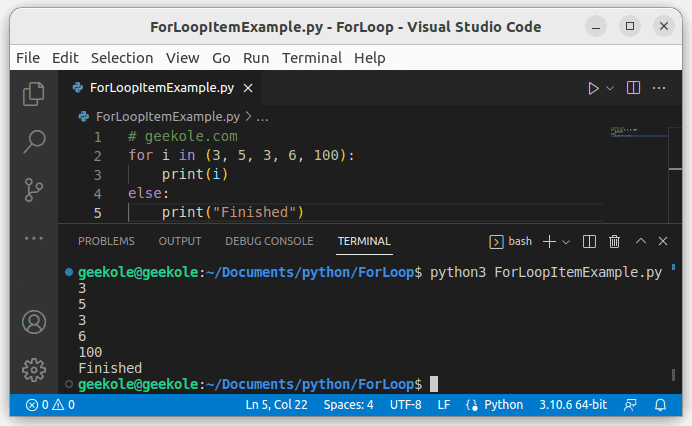
Note: The screenshots in these examples are edited and run on the Visual Studio Code.
We hope this examples of how to use for loop and range() in Python will be useful for you.