Connecting to a server with a Python FTP Client is very easy using the ftplib package. Of course you must have some information at hand to make the connection, such as the server address, have a valid username and password, and finally the port, which is usually 21 for FTP connections.
The connection can be done in one step:
# Connect in one step with FTP(host='ftp.server.com', user='user@server.com', passwd='password') as ftp: print(ftp.getwelcome())
One detail that may be important is that the user value may need to be completed with the details of the server to which you connect, as in:
«user -> user@server.com»
You can also connect in two steps, sending the user data and password on a separate line:
from ftplib import FTP # Connect in separate steps ftp = FTP() ftp.connect('ftp.server.com', 21) ftp.login('user@server.com', 'password') print(ftp.getwelcome()) ftp.close()
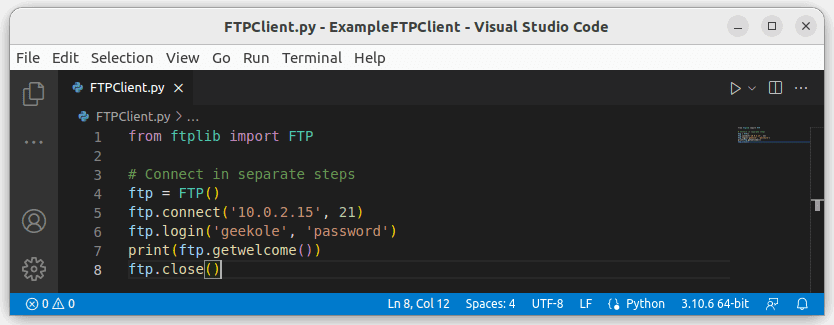
The code block above will display something like the following on the console, but this may vary depending on the FTP server configuration.
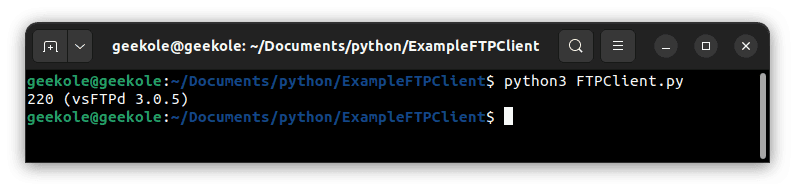
Work with directories
With the function ftp.pwd() you can show the directory you are in:
from ftplib import FTP with FTP('ftp.server.com', 'user', 'password') as ftp: print(ftp.pwd()) # Show default folder
You can create a directory or folder using the ftp.mkd(‘my_directory’) function and passing the directory name as an argument:
from ftplib import FTP with FTP('ftp.server.com', 'user', 'password') as ftp: ftp.mkd('my_directory')
You can remove the directory with the ftp.rmd(‘my_directory’) function using the directory name as an argument:
from ftplib import FTP with FTP('ftp.server.com', 'user', 'password') as ftp: ftp.rmd('my_directory')
To change the active directory you are in you must use ftp.cwd(‘another_directory’) :
from ftplib import FTP with FTP('ftp.server.com', 'user', 'password') as ftp: print(ftp.cwd('another_directory'))
You can list the contents of a directory by first getting an array of files with ftp.dir(files.append) :
from ftplib import FTP with FTP('ftp.server.com', 'user', 'password') as ftp: # generate a list of files files = [] ftp.dir(files.append) for f in files: print(f)
Management of files or archives
There are two ways to send files depending on whether they are text or binary files such as images, for example.
For text you will use the storlines function and for binary files storbinary:
from ftplib import FTP with FTP('ftp.server.com', 'user', 'password') as ftp: # For text or binary file, always use `rb` with open('file.txt', 'rb') as text_file: ftp.storlines('STOR file.txt', text_file) with open('image.png', 'rb') as image_file: ftp.storbinary('STOR image.png', image_file)
To obtain the size of a file or archive, use the size() function, but you must take into account the type of file from which you are obtaining this information, so you must prepare the client to receive information from text files with the value «TYPE A» or for binary files with «TYPE I».
from ftplib import FTP, all_errors with FTP('ftp.server.com', 'user', 'password') as ftp: try: ftp.sendcmd('TYPE I') # For binary files print(ftp.size('image.png')) # get the size of the image on the server except all_errors as error: print(f"Error getting image size: {error}") try: ftp.sendcmd('TYPE A') # For text print(ftp.size('text.txt')) except all_errors as error: print(f"Error getting file size: {error}")
To rename a file, use the rename(‘old_name.txt’, ‘ new_name.txt’) function:
from ftplib import FTP, all_errors with FTP('ftp.server.com', 'user', 'password') as ftp: try: ftp.rename('previous_text.txt', 'new_text.txt') except all_errors as error: print(f'Error renaming file on server: {error}')
And not least, one of the functions that you will surely use the most is downloading files. To do this you will use the retrlines and retrbinary functions, for text and binary files respectively:
from ftplib import FTP, all_errors with FTP('ftp.server.com', 'user', 'password') as ftp: # For text files with open('local_text_name.txt', 'w') as local_file: # Open a text file locally for writing response = ftp.retrlines('RETR text.txt', local_file.write) # Check the answer # https://en.wikipedia.org/wiki/List_of_FTP_server_return_codes if response.startswith('226'): # Full transfer print('Full transfer') else: print('Transfer error. The file may be incomplete or corrupt.') # For binary files with open('local_image.png', 'wb') as local_file: ftp.retrbinary('RETR image.png', local_file.write)
Finally, delete a file, using the delete(”file_name_delete.txt”) function :
from ftplib import FTP, all_errors with FTP('ftp.server.com', 'user', 'password') as ftp: try: ftp.delete('text.txt') except all_errors as error: print(f'Error deleting file: {error}')
It may be useful to know when an FTP command has not been executed successfully, for this you must take into account that the code can throw one of the following errors:
ftplib.error_reply
– unexpected server replyftplib.error_temp
– temporary errorftplib.error_perm
– permanent errorftplib.error_proto
– malformed server replyftplib.all_errors
– catches all above errors that a server can return andOSError
andEOFError
.
from ftplib import FTP with FTP('ftp.server.com', 'user', 'password') as ftp: try: print(ftp.pwd()) except ftplib.all_errors as e: print(f'Error in FTP: {e}')
So far the list of basic functions of the FTP client in Python. We hope these examples of how to create an FTP Client in Python will help you.
You can see more python examples at: https://geekole.com/python/
And the official documentation for ftplib: https://docs.python.org/3/library/ftplib.html