To get information from a file in Python we will use the os module, the path in our storage unit and the following functions:
os.path.getsize()
returns the size of the archive or file.os.path.getmtime()
returns the last modified date of the file.os.path.getctime()
returns the creation date (equal to the last modified date on Unix like Mac).
We show you the code to use these functions:
import os filename = '/home/geekole/Documentos/python/archivos/archivo.txt' print(os.path.getsize(filename)) print(os.path.getmtime(filename)) print(os.path.getctime(filename))
Of course, you must modify the path of the file you are using as an example.
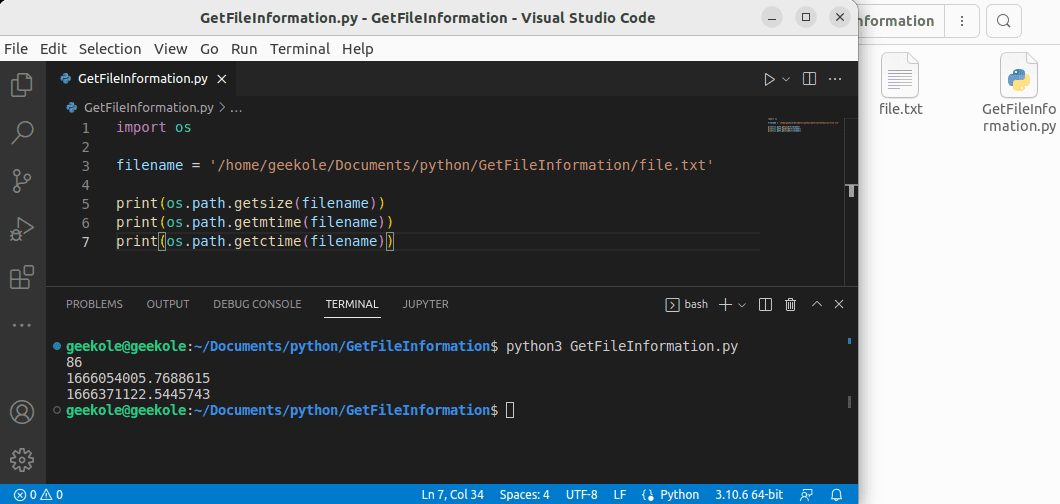
Another way to obtain information in a more concise way is by using the stat() function, which also receives the file path as an argument.
import os filename = '/home/geekole/Documentos/python/archivos/archivo.txt' print(os.stat(filename))
With which you will get something like this:
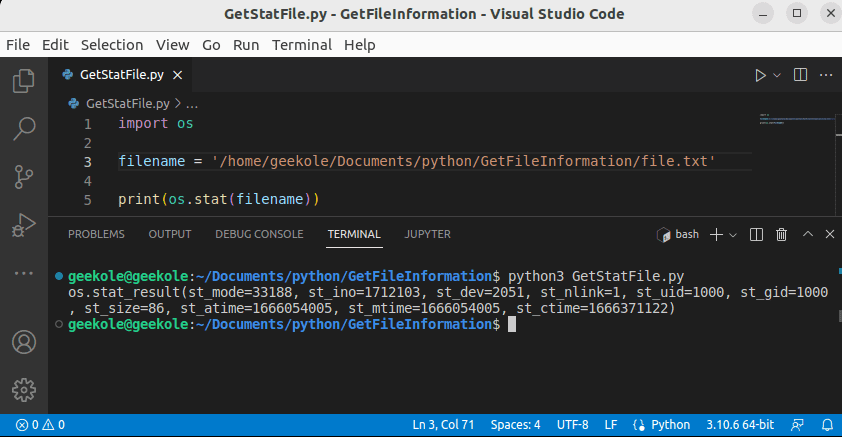
Where each value means the following:
st_mode
the type of file and its permissions.st_ino
the inode number.st_dev
the device id.st_uid
the owner id.st_gid
the group id.st_size
the size of the file.
These properties can be consulted individually:
import os filename = '/home/geekole/Documentos/python/archivos/archivo.txt' stats = os.stat(filename) print(stats.st_size) print(stats.st_mtime)
If you run the above script you will get the following output:
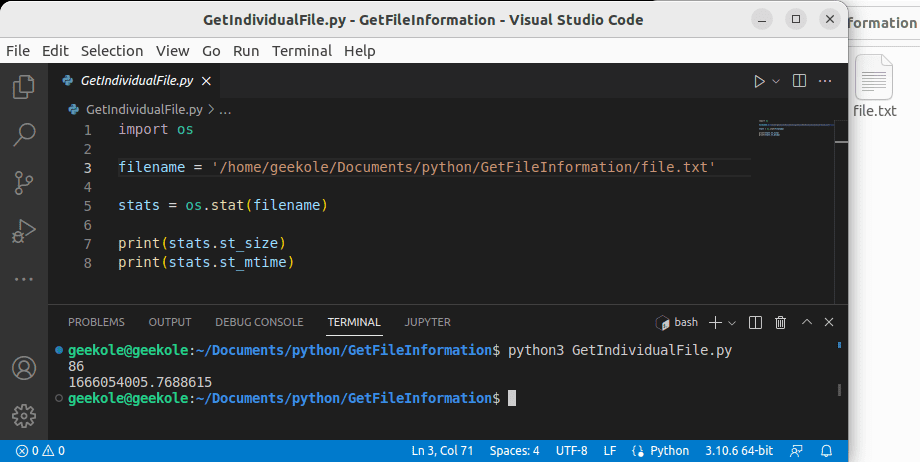
You can also refer to other Python examples for creating and reading text files:
We hope these examples for getting information from a file in Python will be useful to you.
See more information: https://docs.python.org/3/library/os.html