Probably the first example you will do when you learn any programming language is the typical “Hello World”, in Java it’s simple, but unlike other languages like Python, where one line is enough, for Java it takes a little longer.
We show you how to do it:
public class HelloWorld { public static void main(String args[]){ System.out.println("Hello World"); } }
It may seem like a lot of code, but if you follow the structure of this example, “Hello World” will be printed to the console. To see the result you just have to run the compile and run commands in the same path where you save the HelloWorld.java file, of course, make sure you have Java installed previously.
javac HelloWorld.java
java HelloWorld
When you run the first command, the one that calls the compiler, a file called:
HelloWorld.class
When you run the second command, the Java virtual machine will look for the .class extension file with the name of the class you specify and execute it, you don’t need to add the extension. The result is shown in the following image:
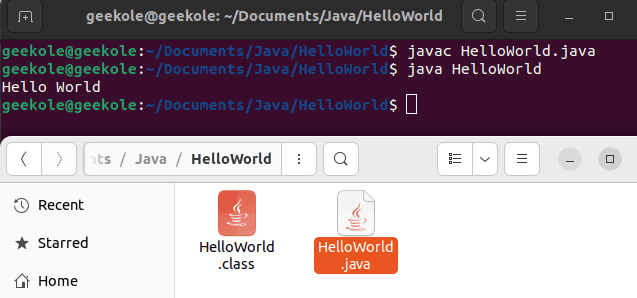
For practical purposes that is all you need to know.
However, if you are curious to know what else happens with this example, we can comment on the following:
- First of all, Java requires for the execution that there is a public method “public”, which can be called externally.
- The method must be “static”, it is not necessary to create an instance of the HelloWorld class
- Must not return “void” values
- The method must be called “main”
- and should be able to receive various arguments in a “String args[]” array specified between the parentheses, where additional commands may be received that may be useful to your program.
When the virtual machine executes a class as shown in the second command, it will look for the main method that meets the characteristics we mentioned above.
The next thing you can notice is the call to the “println()” function, this belongs to an instance of “out” which in turn belongs to the “System” class and is the one that will print the text on the console. At the moment it seems that there is code that doesn’t seem to have a specific function, but it does. When you advance in the knowledge of the language it will be easier to understand the reason.
We hope this example of Hello World in Java will be useful to you.