Json is a widely used lightweight text format for data exchange on the Web, it is supposed to be a replacement for XML as it is more compact and easier to read. In this example we will convert a Java object with information to a text string in Json format with the help of the Google Gson library.
We import the dependency into Maven:
<dependency> <groupId>com.google.code.gson</groupId> <artifactId>gson</artifactId> <version>2.8.5</version> </dependency>
The code is the following:
package com.geekole; // Name of your java package import com.google.gson.Gson; import java.io.FileWriter; /** * * @author geekole.com */ public class JsonWithGson { public static void main(String args[]){ try { CompanyData companyData = new CompanyData(); companyData.setId(1); companyData.setName("Auto parts distributor"); companyData.setAddress("Central Street No. 111"); Gson gson = new Gson(); String stringJson = gson.toJson(companyData); System.out.println("companyData Json: " + stringJson); FileWriter fileWriter = new FileWriter("/path/jsonFile.json"); fileWriter.write(stringJson); fileWriter.close(); } catch (Exception e) { e.printStackTrace(); } } }
Additionally we use a CompanyData class that serves as an example to convert to Json format.
package com.geekole; // Name of your java package /** * * @author geekole.com */ public class CompanyData { private long id; private String name; private String address; private boolean status; public long getId() { return id; } public void setId(long id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } public boolean isStatus() { return status; } public void setStatus(boolean status) { this.status = status; } }
We convert our CompanyData instance to a Json string that can be used to respond to a request in the case of a servlet or to be stored in a text file depending on your need.
There really isn’t much to do, we just use the gson.toJson(companyData) method and convert our companyData object to a string.
Gson gson = new Gson(); String stringJson = gson.toJson(companyData);
Running the example we get the following output:
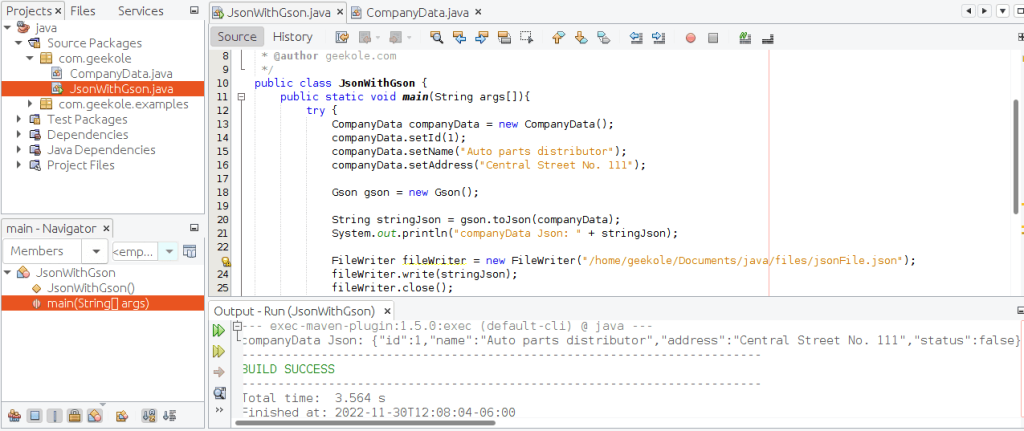
Additionally, we save our Json object in a fileJson.json file:
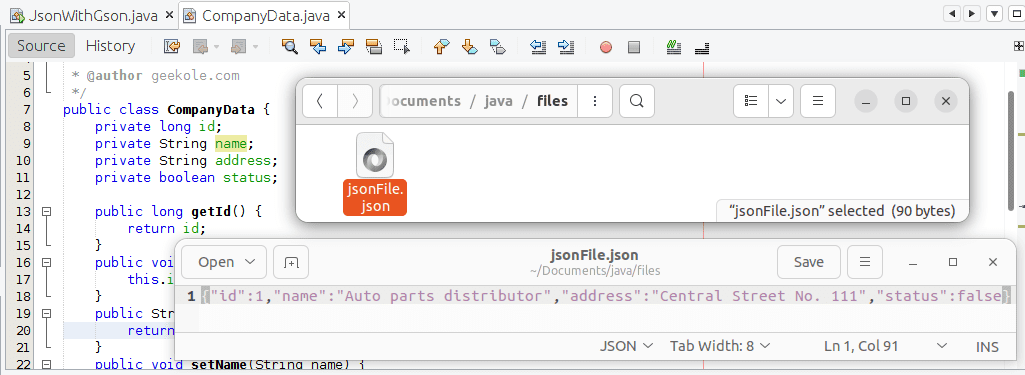
And that’s it. We hope that this example of Json with Google Gson in Java will be useful to you.