To list and filter files with FileFilter in Java we must import File and FileFilter from the java.io package. Overwriting the accept function of FileFilter we can define the characteristics, prefixes, suffixes or the file extension that we want to filter.
We show you the code:
package com.geekole; // Name of your java package import java.io.File; import java.io.FileFilter; /** * * @author geekole.com */ public class FilterFilesWithFilefilter { public static void main(String[] args) { //We create our file filter FileFilter filesFilter = new FileFilter() { //We override the method public boolean accept(File file) { if (file.getName().endsWith(".txt")) { return true; } return false; } }; File directory = new File("/path/"); //We check if the folder exists if (!directory.exists()) { System.out.println(String.format("The folder %s does not exist.", directory.getName())); return; } //We verify that it is a folder or directory and not a file if (!directory.isDirectory()) { System.out.println(String.format("Entry %s is not a directory", directory.getName())); return; } File[] files = directory.listFiles(filesFilter); //We list in a for loop the results for (File f : files) { System.out.println(f.getName()); } } }
As you can see, we instantiate and override the FileFilter class and its accept() method and all we do is return a true value if the filename string ends with a “.txt” extension.
FileFilter filesFilter = new FileFilter() { //We override the method public boolean accept(File file) { if (file.getName().endsWith(".txt")) { return true; } return false; } };
With String functions you can search for a specific string, a prefix, or you can add more conditions to include other file extensions.
The following lines simply create an instance of the File class with the directory path of interest. We verify that it exists and that it is indeed a directory.
File directory = new File("/path/"); //We check if the folder exists if (!directory.exists()) { System.out.println(String.format("The folder %s does not exist.", directory.getName())); return; } //We verify that it is a folder or directory and not a file if (!directory.isDirectory()) { System.out.println(String.format("Entry %s is not a directory", directory.getName())); return; }
Later we use the File function called listFiles(), which returns an array of File with the files found. This function receives as an argument the variable filesFilter that we created at the beginning.
File[] files = directory.listFiles(filesFilter); //We list in a for loop the results for (File f : files) { System.out.println(f.getName()); }
Run the example code
In our example we are looking for files in a directory where at least one of them does not meet our condition:
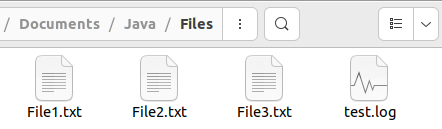
If you run the example you will get the list of files that match our condition in FileFiter:
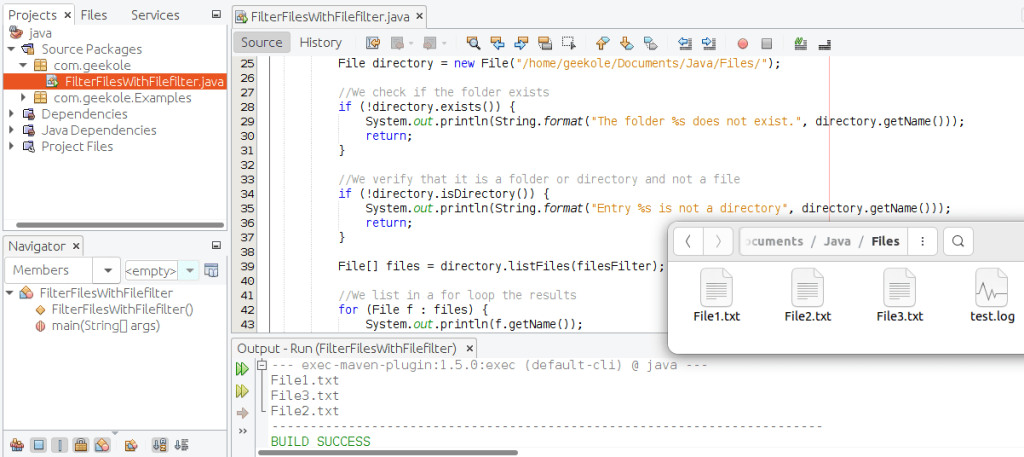
In the output window of our editor, the file «test.log» has been omitted from the results list. Another thing that you should observe is that it may happen that the list is displayed in a different order than the one we see in the file explorer, the ordering in the data output that you show to the user will depend on you and you must take into account the options that each element of the File array has available as name, date or size.
We hope that this example to List and Filter files with FileFilter in Java will be useful to you.
See documentation: https://docs.oracle.com/javase/8/docs/api/java/io/FileFilter.html