To create a simple PDF with Python we will use ReportLab, which is one of the most widely used libraries. The code for this example is simple:
import time from reportlab.lib.enums import TA_JUSTIFY from reportlab.lib.pagesizes import letter from reportlab.platypus import SimpleDocTemplate, Paragraph, Spacer, Image from reportlab.lib.styles import getSampleStyleSheet, ParagraphStyle from reportlab.lib.units import inch # geekole.com doc = SimpleDocTemplate("/path/test.pdf", pagesize=letter, rightMargin=72, leftMargin=72, topMargin=72, bottomMargin=18) Story = [] logo = "/path/python_logo.png" magaziNename = "Programation Advance" number = 4 price = "10.00" deadline = "27/09/2017" gift = "Python workshop" dateFormat = time.ctime() fullName = "Antony Smith" addressParts = ["Street with number 123", "Suburb, Postal Code 12345"] image = Image(logo, 1 * inch, 1 * inch) Story.append(image) styles = getSampleStyleSheet() styles.add(ParagraphStyle(name='Justify', alignment=TA_JUSTIFY)) text = '%s' % dateFormat Story.append(Paragraph(text, styles["Normal"])) Story.append(Spacer(1, 12)) # Address is built text = '%s' % fullName Story.append(Paragraph(text, styles["Normal"])) for part in addressParts: text = '%s' % part.strip() Story.append(Paragraph(text, styles["Normal"])) Story.append(Spacer(1, 12)) text = 'Dear %s:' % fullName.split()[0].strip() Story.append(Paragraph(text, styles["Normal"])) Story.append(Spacer(1, 12)) text = 'We would like to welcome you as a subscriber to our magazine %s ! \ Will receive %s numbers with an introductory price of $%s. Please reply before of \ %s to start receiving your subscription and also get your gift: %s.' % (magaziNename, number, price, deadline, gift) Story.append(Paragraph(text, styles["Justify"])) Story.append(Spacer(1, 12)) text = 'Thank you and we hope to have served you.' Story.append(Paragraph(text, styles["Justify"])) Story.append(Spacer(1, 12)) text = 'To be honest,' Story.append(Paragraph(text, styles["Normal"])) Story.append(Spacer(1, 48)) text = 'Oliva Pattinson' Story.append(Paragraph(text, styles["Normal"])) Story.append(Spacer(1, 12)) doc.build(Story)
This example makes some statements that are important. First, the type of document that we will use is declared and created:
doc = SimpleDocTemplate("/path/test.pdf", pagesize=letter, rightMargin=72, leftMargin=72, topMargin=72, bottomMargin=18)
In the previous lines of code we specified the name of the PDF files that we are going to create and their dimensions.
We also declare the path of an image in png format (a square with transparency of 180×180 pixels) of which we will later define the position and dimensions within the document.
logo = "/path/python_logo.png"
image = Image(logo, 1 * inch, 1 * inch) Story.append(image)
We also dynamically declare and use at least two styles for the text. “Normal” that comes by default and one that we add dynamically called “Justify”.
styles = getSampleStyleSheet() styles.add(ParagraphStyle(name='Justify', alignment=TA_JUSTIFY))
You can see that the content of our document is gradually being built with the help of the Story variable.
Story.append(image) . Story.append(Paragraph(text, styles["Normal"])) Story.append(Spacer(1, 12))
We are adding paragraphs, images or simple spaces.
Finally we use “build” to generate our document:
doc.build(Story)
And you should get something like this:
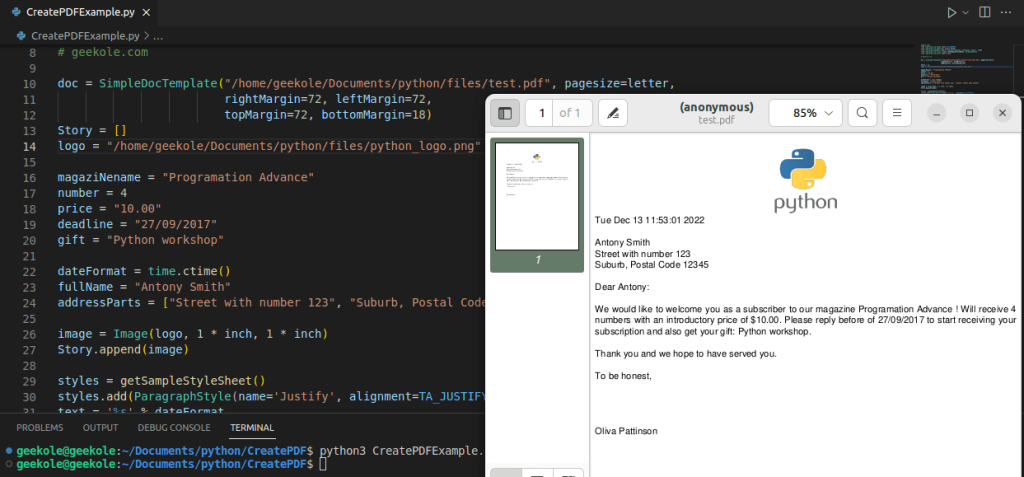
However, when you copy the code, don’t forget to change the path of the PDF file to be generated and the path of the image, which you can change to another one of a similar size but with the same extension. We hope this simple example of a PDF with ReportLab in Python will be useful for you to build more elaborate documents.
More information at: http://www.reportlab.com