To read a text file in Java, there are several ways to do it, but one of the easiest is to use the Scanner class, as shown below:
package com.geekole; // Name of your java package import java.io.File; import java.util.Scanner; /** * @author geekole.com */ public class ReadFile { public static void main(String[] args) { try { Scanner input = new Scanner(new File("/path/filename.txt")); while (input.hasNextLine()) { String line = input.nextLine(); System.out.println(line); } input.close(); } catch (Exception ex) { ex.printStackTrace(); } } }
Using the hasNextLine() method you can determine if the text file contains lines that can be read, normally this is done within a loop on the stop condition, and then read the contents of them with the nextLine() method, when the hasNextLine() method stops returning true the read loop should stop. It is important that after reading the information you need from the file, you use the close() method, which will release the resource so that it can be used without problems later.
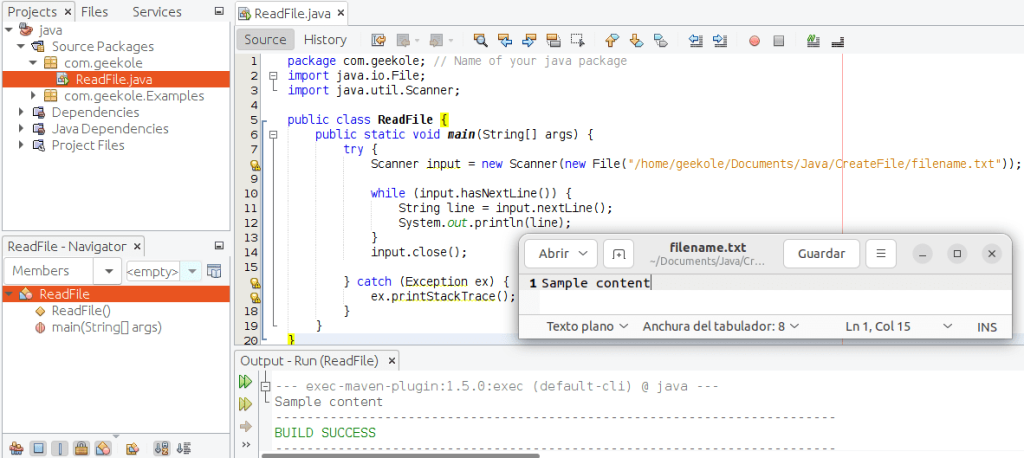
We hope this example of read a text file in Java will help you.