To read an Excel file with Apache in Java an .xlsx extension, we use org.apache.poi whose Maven dependency is as follows.
<dependency> <groupId>org.apache.poi</groupId> <artifactId>poi-ooxml</artifactId> <version>3.16</version> </dependency>
This will be the excel file that we will read.
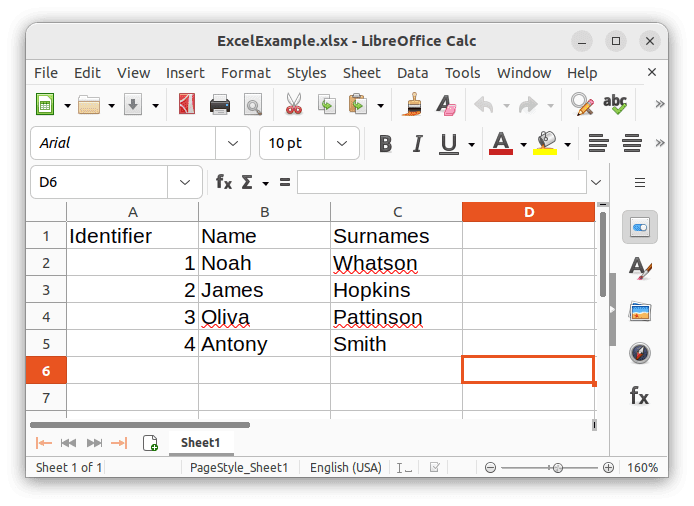
The code:
package com.geekole; // Name of your java package import java.io.File; import java.io.FileInputStream; import java.util.Iterator; import org.apache.poi.ss.usermodel.Cell; import org.apache.poi.ss.usermodel.Sheet; import org.apache.poi.ss.usermodel.DataFormatter; import org.apache.poi.ss.usermodel.Row; import org.apache.poi.ss.usermodel.Workbook; import org.apache.poi.xssf.usermodel.XSSFWorkbook; /** * * @author geekole.com */ public class ReadExcel { public static void main(String args[]){ try { String pathExcelFile = "/path/ExcelExample.xlsx"; FileInputStream inputStream = new FileInputStream(new File(pathExcelFile)); Workbook workbook = new XSSFWorkbook(inputStream); Sheet firstSheet = workbook.getSheetAt(0); Iterator<Row> iterator = firstSheet.iterator(); DataFormatter formatter = new DataFormatter(); while (iterator.hasNext()) { Row nextRow = iterator.next(); Iterator<Cell> cellIterator = nextRow.cellIterator(); while(cellIterator.hasNext()) { Cell cell = cellIterator.next(); String cellContent = formatter.formatCellValue(cell); System.out.println("cell: " + cellContent); } } } catch (Exception e) { e.printStackTrace(); } } }
Next we show the most interesting parts of the code, with XSSFWorkbook and a FileInputStream we start reading the file.
Workbook workbook = new XSSFWorkbook(inputStream);
Our workbook instance allows us to read an excel sheet, indicating the index in which the information is found. In this single sheet example we specify index zero.
Sheet firstSheet = workbook.getSheetAt(0);
Next we begin to iterate over each row or row that the excel sheet contains.
Iterator iterator = firstSheet.iterator();
while (iterator.hasNext()) { …
Then we iterate over the row to get its cells.
Iterator cellIterator = nextRow.cellIterator();
while(cellIterator.hasNext()) { …
When obtaining the value of each cell, we are also formatting it to be able to display it as a string, otherwise we have to obtain the data type of each cell before using the specific method that allows us to extract its value according to its type. With this step we skip that previous validation.
String contenidoCelda = formatter.formatCellValue(cell);
If we run the example we get this result:
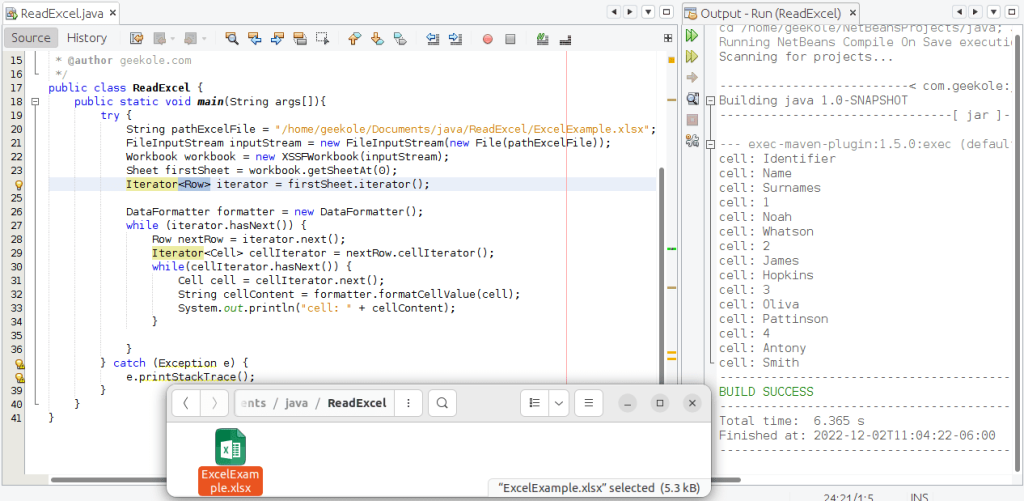
The example to Read an Excel file with Apache in Java is very simple, but it will allow you to easily start reading Excel files.