Read XML file in Python is very easy, the file that we will use for reading in this example is the following:
data.xml
<?xml version="1.0"?> <company> <employee id="1"> <name>Jackson Aiden</name> <username>jackson</username> <password>321423</password> </employee> <employee id="2"> <name>Emma Sophia</name> <username>emma</username> <password>433543</password> </employee> </company>
We import minidom.
from xml.dom import minidom doc = minidom.parse("/path/data.xml") name = doc.getElementsByTagName("name")[0] print(name.firstChild.data) employees = doc.getElementsByTagName("employee") for employee in employees: sid = employee.getAttribute("id") username = employee.getElementsByTagName("username")[0] password = employee.getElementsByTagName("password")[0] print("id:%s " % sid) print("username:%s" % username.firstChild.data) print("password:%s" % password.firstChild.data)
To start the parsing we use: doc = minidom.parse(“/path/data.xml”).
It is possible to find any node of the document as we do in the line with the function name = doc.getElementsByTagName(“name”)[0].
Get a list of nodes and their attributes: sid = employee.getAttribute(“id”).
Or get other nodes and their respective text content: username = employee.getElementsByTagName(“username”)[0].
With which you will get something like this:
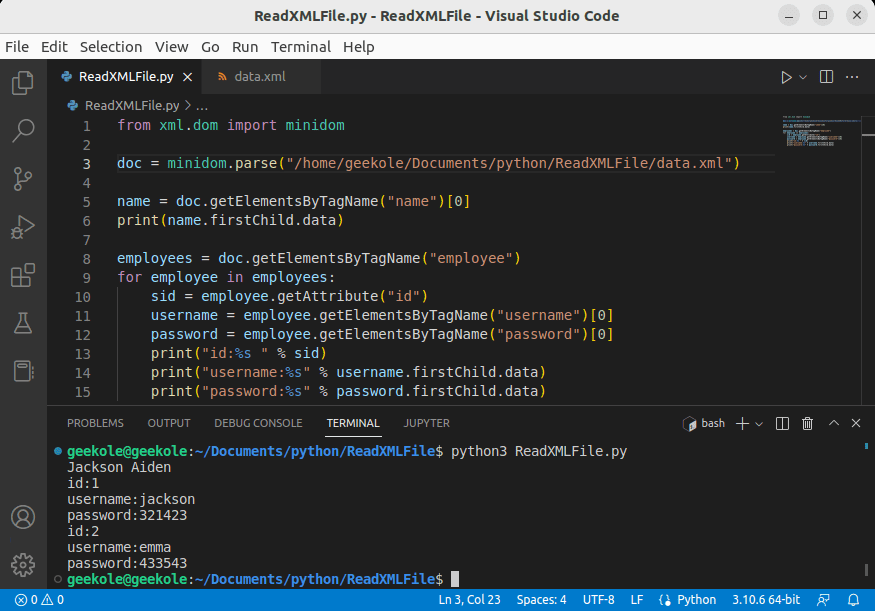
We hope this example of read an XML file in Python will help you.