In this example, we will show how to read an XML with SAX Parser in Java, one of the most efficient options for reading XMLs and one that uses less memory.
For this example we use an XML with the following structure:
data.xml
<?xml version="1.0"?> <company> <employee id="1"> <name>Jackson Aiden</name> <username>jackson</username> <password>321423</password> </employee> <employee id="2"> <name>Emma Sophia</name> <username>emma</username> <password>433543</password> </employee> <employee id="3"> <name>Tom Michael</name> <username>Tom</username> <password>534534</password> </employee> </company>
Let’s go to the code:
package com.geekole; // Name of your java package import java.io.File; import java.io.FileInputStream; import java.io.InputStream; import java.io.InputStreamReader; import java.io.Reader; import javax.xml.parsers.SAXParser; import javax.xml.parsers.SAXParserFactory; import org.xml.sax.Attributes; import org.xml.sax.InputSource; import org.xml.sax.SAXException; import org.xml.sax.helpers.DefaultHandler; /** * * @author geekole.com */ public class ReadXMLWithSAX { public static void main(String argv[]) { try { SAXParserFactory factory = SAXParserFactory.newInstance(); SAXParser saxParser = factory.newSAXParser(); DefaultHandler handler = new DefaultHandler() { boolean bName = false; boolean bUsername = false; boolean bPassword = false; public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException { System.out.println("start element:" + qName); if (qName.equalsIgnoreCase("employee")) { String id = attributes.getValue("id"); System.out.println("id: " + id); } if (qName.equalsIgnoreCase("name")) { bName = true; } if (qName.equalsIgnoreCase("username")) { bUsername = true; } if (qName.equalsIgnoreCase("password")) { bUsername = true; } } public void endElement(String uri, String localName, String qName) throws SAXException { System.out.println("ends element:" + qName); } public void characters(char ch[], int start, int length) throws SAXException { if (bName) { System.out.println("name: " + new String(ch, start, length)); bName = false; } if (bUsername) { System.out.println("username: " + new String(ch, start, length)); bUsername = false; } if (bPassword) { System.out.println("password: " + new String(ch, start, length)); bPassword = false; } } }; File file = new File("/path/data.xml"); InputStream inputStream = new FileInputStream(file); Reader reader = new InputStreamReader(inputStream, "UTF-8"); InputSource is = new InputSource(reader); is.setEncoding("UTF-8"); saxParser.parse(is, handler); } catch (Exception e) { e.printStackTrace(); } } }
The first thing you should notice is that we are building a «handler», which is in charge of reading and registering specific parts of our XML. It is necessary to clarify that we must know the structure of the XML file that we are reading.
While the file is being read, we are discovering elements of interest in our XML which we register with the help of boolean variables:
boolean bName = false; boolean bUsername = false; boolean bPassword = false;
With the help of the startElement method we register each encounter and change the value of our boolean variables.
public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException { System.out.println("start element:" + qName); if (qName.equalsIgnoreCase("employee")) { String id = attributes.getValue("id"); System.out.println("id: " + id); } if (qName.equalsIgnoreCase("name")) { bName = true; } if (qName.equalsIgnoreCase("username")) { bUsername = true; } if (qName.equalsIgnoreCase("password")) { bUsername = true; } }
The characters method will allow us to obtain the information contained in the XML tag found. After doing «something» with the information found (in our case we just print it to the console), we return to the state before the boolean that corresponds to the XML tag.
public void characters(char ch[], int start, int length) throws SAXException { if (bName) { System.out.println("name: " + new String(ch, start, length)); bName = false; } if (bUsername) { System.out.println("username: " + new String(ch, start, length)); bUsername = false; } if (bPassword) { System.out.println("password: " + new String(ch, start, length)); bPassword = false; } }
If you run the code you will see in the console that every time a name, username or password label is found, its content is printed:
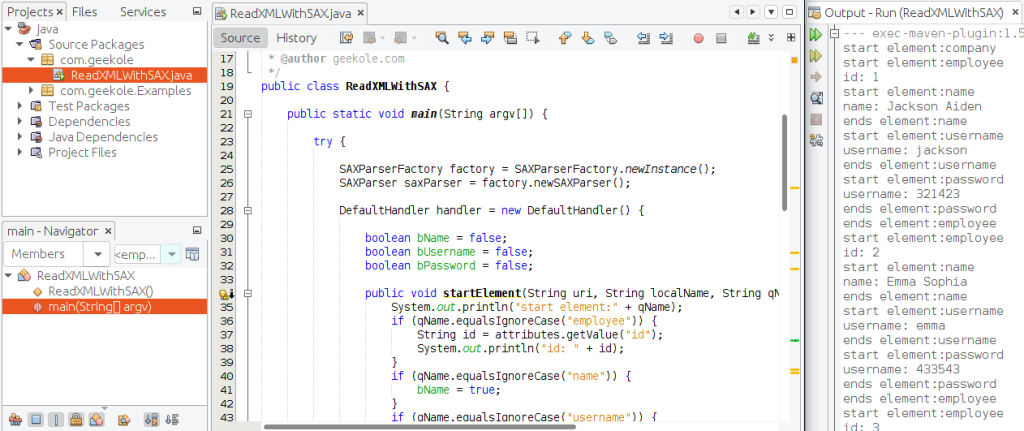
To read the attributes of a label, we use the attributes variable that we receive as an argument in the startElement method and we simply take their value with the getValue function.
String id = attributes.getValue("id");
Finally we show you how to initialize the parser using the parse method, which receives the data from the file and the handler that we implement specifically for the example XML structure.
File file = new File("/path/data.xml"); InputStream inputStream = new FileInputStream(file); Reader reader = new InputStreamReader(inputStream, "UTF-8"); InputSource is = new InputSource(reader); is.setEncoding("UTF-8"); saxParser.parse(is, handler);
The parse method can directly receive a file variable and the handler, but we do it this way so we can specify that the file to be read contains UTF-8 characters.
We hope this example of read XML with SAX Parser will be useful to you.