In Java, regular expressions are checked with the help of the Pattern and Matcher classes. Here’s an example that lets you check if a string matches the pattern of an email address.
package com.geekole; // Name of your java package import java.util.regex.Matcher; import java.util.regex.Pattern; /** * * @author geekole.com */ public class ExampleRegularExpression { private static final String PATTERN_EMAIL = "^[_A-Za-z0-9-\\+]+(\\.[_A-Za-z0-9-]+)*@" + "[A-Za-z0-9-]+(\\.[A-Za-z0-9]+)*(\\.[A-Za-z]{2,})$"; public static void main(String args[]){ String mailAddress = "test_mail@domain.com"; Pattern pattern = Pattern.compile(PATTERN_EMAIL); Matcher matcher = pattern.matcher(mailAddress); boolean result = matcher.matches(); System.out.println("result: "+ result); } }
In the java example, it is necessary to declare the regular expressions that will be verified in a string, we do this with the constant PATTERN_EMAIL.
Later the pattern is “compiled” with the help of Pattern and the compile method, we use the matcher method from which we obtain an instance of Matcher, which will tell us what is the result of the verification of the expression on the string mailAddress.
If you run the example you will get this output:
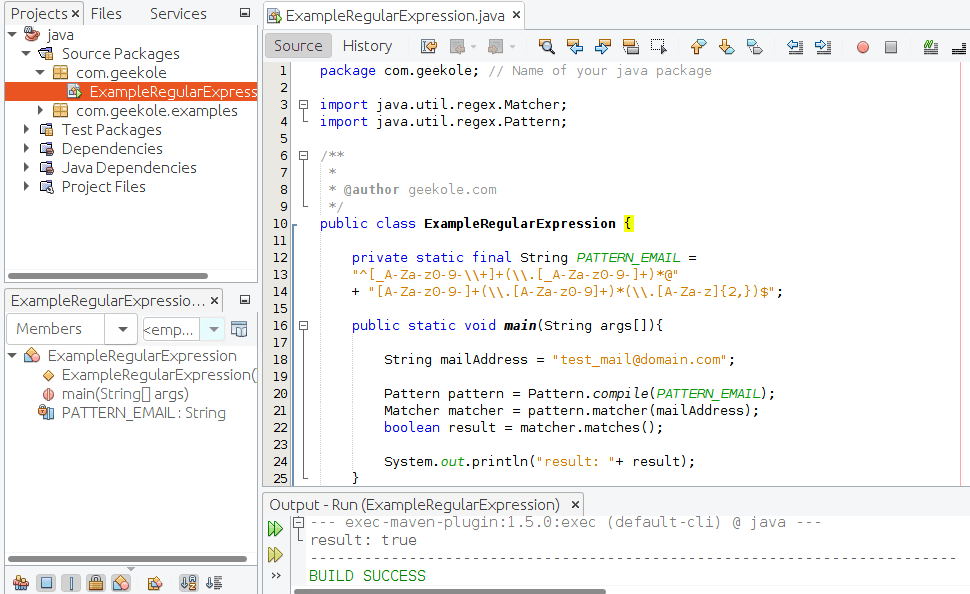
As you can see in the lower part of the image, the result is that our email address complies with the expression, returning true.
There are different types of regular expressions. Here are other examples:
String PATRON_USERNAME = "^[a-z0-9_-]{3,15}$"; String PATRON_PASSWORD = "((?=.*\d)(?=.*[a-z])(?=.*[A-Z])(?=.*[@#$%]).{6,20})"; String PATRON_IMAGE_EXTENSION = "([^\s]+(\.(?i)(jpg|png|gif|bmp))$)";
Note: the examples are edited and run in NetBeans.