In Java, as in many programming languages, there is an IF statement. The if statement is a control statement that allows you to execute blocks of code depending on a condition. The basic structure of this statement and its syntax is as follows:
if (condition) { sentences }
A slightly more real and executable example is the following:
package com.geekole; // Name of your java package public class IFExample { public static void main(String args[]){ if (true) System.out.println("First example of IF"); if (true) { System.out.println("Second example of IF"); } } }
In the previous lines of code we have an if with a single statement and later an if with a block of code that can have one or more statements. In the second example, delimited by the square brackets “{}” are the “statements” that must be executed if the “condition” is fulfilled.
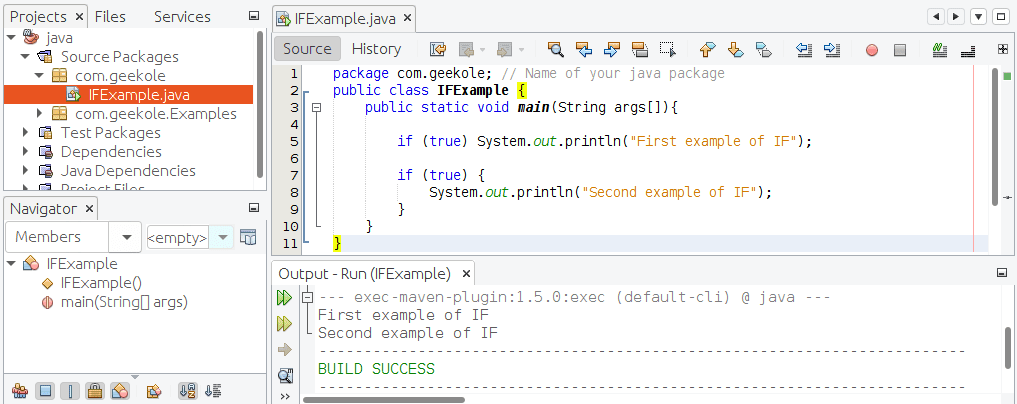
However, these examples are perhaps too simple. Normally, the condition involves the use of one or more variables and of course more statements in the code block. Sometimes it will be necessary to make comparisons to return a true or false Boolean value in the condition, as in the following example:
package com.geekole; // Name of your java package public class IFExample { public static void main(String args[]){ int i = 1; int j = 2; if (i < j) { System.out.println("IF Example"); } } }
Executing the above code will result in the following in the console:
IF Example
You can make the example.
IF ELSE Sentence
Of course, there is also the IF ELSE statement. Which allows you to execute another block of code, when the first condition is not met, and to do the evaluation of another condition immediately after the first one is not met.
package com.geekole; // Name of your java package public class IFExample { public static void main(String args[]){ int i = 3; int j = 1; int k = 2; if (i < j) { System.out.println("The first condition is met."); } else if (i < k) { System.out.println("The first condition is not met."); } else { System.out.println("The second condition is not met."); } } }
The result will be the following:
The second condition is not met.
None of the conditions is met.
Nested IF statements.
It is completely normal to have an if within a code block of another if statement.
package com.geekole; // Name of your java package public class IFExample { public static void main(String args[]){ int i = 3; int j = 1; int k = 2; if (i > j) { System.out.println("The first condition is met."); if (k < i) { System.out.println("The nested if condition is met."); if (j < k) { System.out.println("The condition of the second nested if is met."); } } } else { System.out.println("The first condition is not met."); } } }
The only thing you have to take care of is the congruence in the evaluation of each condition, that is to say, it would not make sense to insert an if in a condition in an else that has not been fulfilled in the condition previously.
package com.geekole; // Name of your java package public class IFExample { public static void main(String args[]){ int i = 2; int j = 1; if (i < j) { System.out.println("The first condition is met."); } else { if(i < j) { System.out.println("This message will never be displayed."); } } } }
In the example above, there is one line of code that will never be displayed: “This message will never be displayed.”
One thing to note is that you will probably do different types of comparisons when checking a condition, especially if you are not evaluating on the direct value of a boolean variable. For these comparisons you will use the different operators that exist such as equal, different from, less than or greater than, etc. We’ll see examples of the IF statement in Java another time.