The ternary operator in Python, as in other languages, has the purpose of making an If condition more compact, allowing conditional code to be generated in a single line. This operator is common in several languages and although it is not widely used it does have its advantages. It has the following general form:
# # [code if met] if [condition] else [code if not met] #
In a normal If statement we would have something like this:
# geekole.com value = 10 if value == 10: print("The value is 10") else: print("The value is 20")
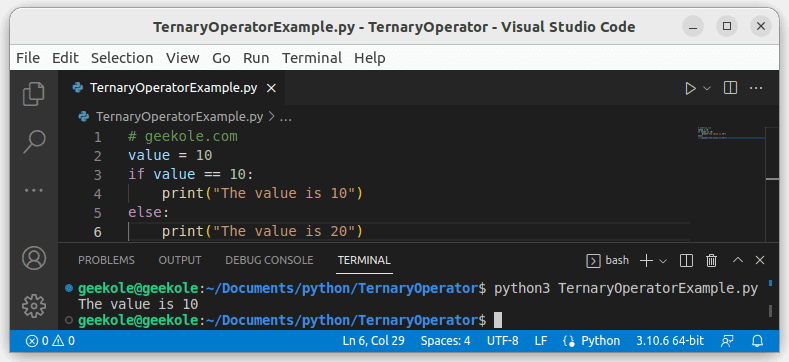
As you can see, it takes at least two lines to print one of the two strings. With the ternary operator we could reduce the code with the same result as follows:
# geekole.com value = 10 print("The value is 10" if value == 10 else "The value is 20")
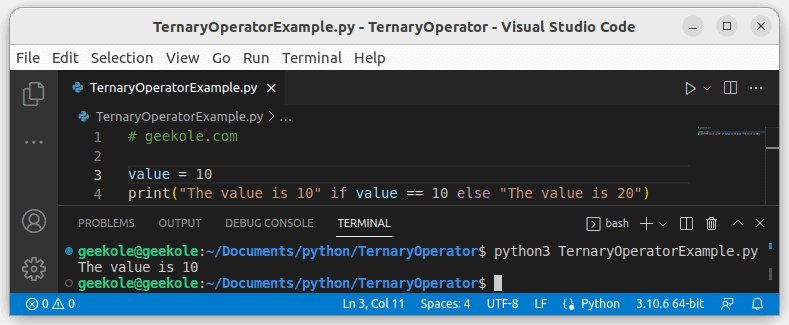
It is also possible to make assignments and operations that are determined by the result of the condition after the If.
# geekole.com value = True a = 100 b = 1000 i = a/10 if value else b/10 print(i)
The console output of this example will be as follows:
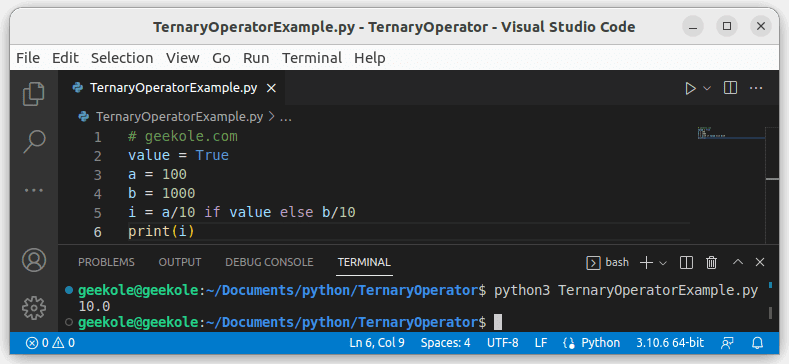
If the value of the condition is true, divide “a” by ten, and if it is false, divide “b” by ten. The condition could be “value == True” to be more explicit, but the idea is to compact the code.
This is how the ternary operator works in Python, its use is almost due to programming style, some consider that it can be less readable and seem impractical if the code is part of a project where more than one programmer maintains and updates the code.
Note: The examples are edited and run with the help of Visual Studio Code.
We hope this example of how to use the Ternary Operator in Python will be useful for you.
More detail at: https://docs.python.org/es/3/reference/expressions.html