The while loop in Java allows a block of code to be executed as long as a specified condition is true, the basic structure is as follows:
while (condition) { //Code to be executed }
In the following example, the loop will be executed over and over again, as long as the variable “i” is less than 10:
package com.geekole; // Name of your java package public class WhileExample { public static void main(String args[]) { int i = 0; while (i < 10) { System.out.println("i: " + i); i++; } } }
You should notice that the variable “i” is assigned the value zero before reaching the while loop, the increment of the value of this variable is done inside the code block of the loop with the help of the ++ operator, which increments the value of this variable. value by one on each pass. When executing the code you will get a result like the following:
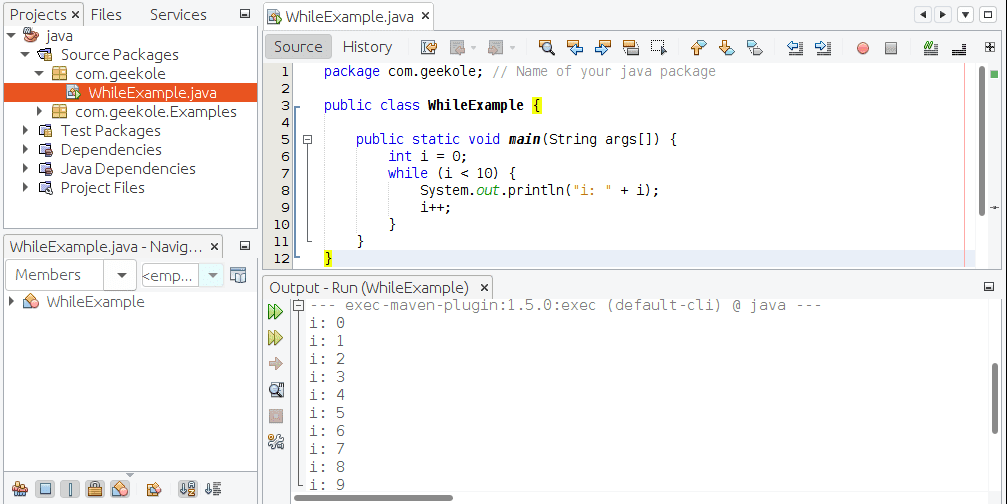
The do-while loop
Another variant of the while loop is the do-while loop. This loop will execute the block of code at least once, before checking if the condition is true.
do { //Code to be executed } while (condition);
The following example uses a do-while loop.
package com.geekole; // Name of your java package public class WhileExample { public static void main(String args[]) { int i = 0; do { System.out.println(i); i++; } while (i < 10); } }
This variant of the loop is useful if you want your code to be executed at least once, before checking that the condition is true and even if the condition is false and terminates immediately.
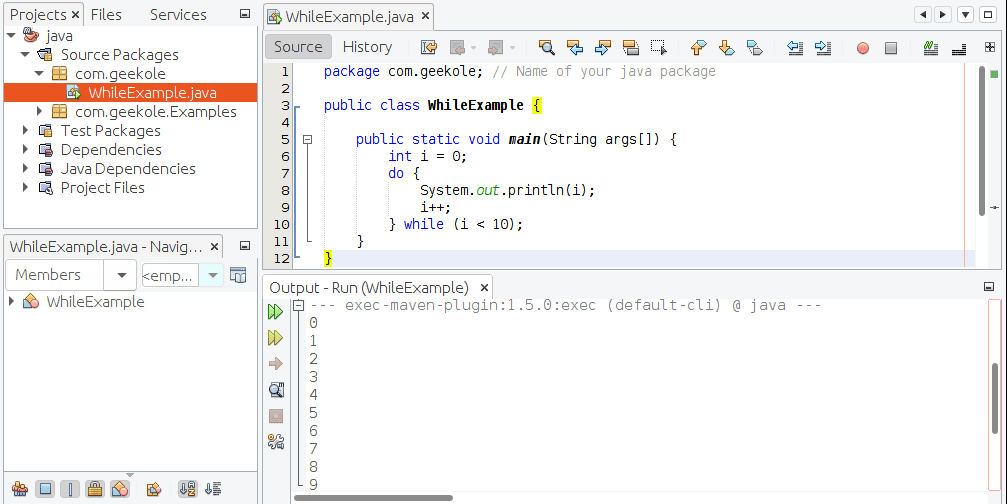
We hope these examples of the while loop in Java will help you.