The while loop is very simple in Python. With the while loop we can execute a set of statements as long as a condition is true.
# geekole.com i = 1 while i < 10: print(i) i += 1
Unlike the for statement, the variable with which we control the number of iterations is usually initialized before and outside of the while, but this depends a lot on how you use the statement in your code.
The example that we show you, only prints a sequence from 1 to 9.

Of course, the while loop supports the use of the break and continue statements.
While and break
# geekole.com i = 1 while i < 10: print(i) if i == 5: break i += 1
This example prints a sequence from 1 to 5, the break statement breaks the remaining iterations.
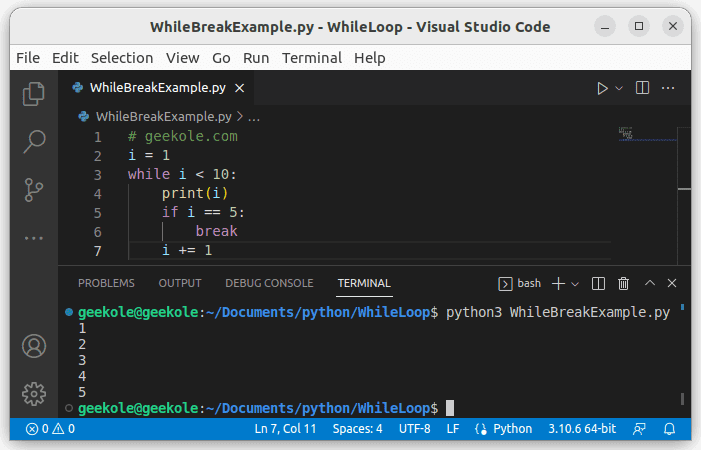
While and continue
# geekole.com i = 1 while i < 10: i += 1 if i == 5: continue print(i)
This example skips printing the number 5, the continue statement interrupts the current iteration, and nothing is printed until the next iteration.

We hope these examples of how to use the while loop in Python make it clear to you how the while function works.
Note: The examples are edited and run with the help of Visual Studio Code.