Create a graphic interface with JavaFX is easy. This library allows you to design, build, test, and deploy cross-platform GUI applications. With JavaFX you can create windows or graphical user interfaces for desktop applications. It is intended to replace Swing as the standard GUI library for Java. With the advent of new operating systems and much higher resolution monitors, Swing has fallen far behind.
JavaFX Features
The following are the key features of JavaFX that make it a good candidate for any desktop application development:
- It comes with a lot of interface components like TableView, ListView, WebView, Form Controls, DatePicker, etc.
- All UI components in JavaFx can be styled using CSS.
- You can use FXML, an XML-based language, to create the user interface of your application.
- The JavaFX Scene Builder tool allows you to design user interfaces without the need to write code. It contains a WYSIWYG drag and drop interface by which the GUI layout can be created. This tool can be integrated into all major IDEs like Netbeans, IntelliJ, and Eclipse.
- It has 3D graphics features to create shapes like Box, Cylinder, Sphere, etc.
- You can create native installable packages of JavaFX applications for all major operating systems. These packages provide the same launch experience as any native application for that operating system.
We show you a simple example:
package com.geekole; // Name of your java package import javafx.application.Application; import javafx.geometry.Pos; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.stage.Stage; /** * * @author geekole.com */ public class JavaFXExample extends Application { @Override public void init() throws Exception { super.init(); System.out.println("Perform the necessary initializations here."); } @Override public void start(Stage primaryStage) throws Exception { Label label = new Label("Hello World"); label.setAlignment(Pos.CENTER); Scene scene = new Scene(label, 500, 350); primaryStage.setTitle("Hello World application"); primaryStage.setScene(scene); primaryStage.show(); } @Override public void stop() throws Exception { super.stop(); System.out.println("Destroy the resources. Perform cleaning."); } public static void main(String[] args) { launch(args); } }
The main class of any JavaFX application must extend the javafx.application.Application class and implement its start() method. The start() method is the starting point for the execution of a JavaFX application.
If you run the example a simple Hello World in a small window will be displayed on your screen.
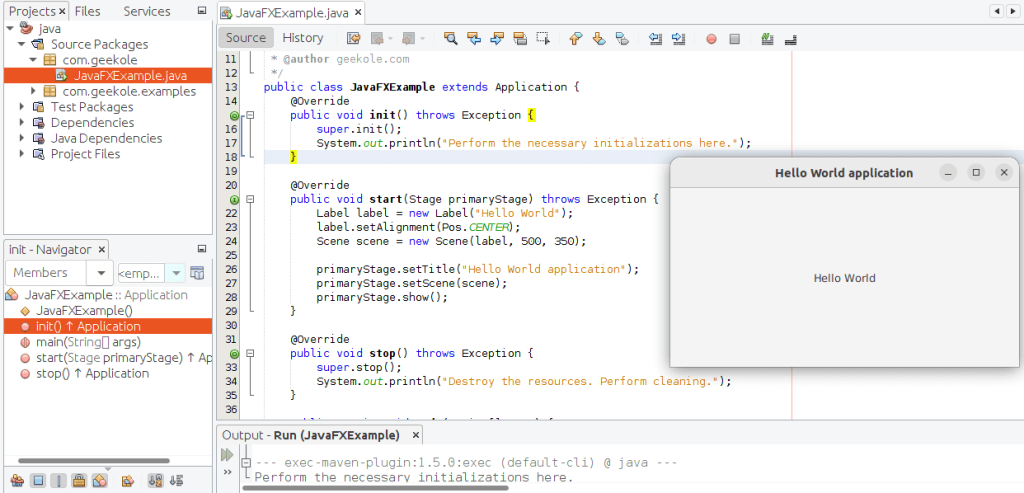
If you are on Linux you will probably get this error:

So you have to run the following command in the terminal to download the libcanberra-gtk module and solve it:
sudo apt install libcanberra-gtk-module libcanberra-gtk3-module
If you are on Linux you should probably run this command in terminal to download the libcanberra-gtk module
As you can see, this is just a simple example of how to create a graphic interface with JavaFX. Soon we will add more examples with components that allow the user to view or enter data such as text boxes and buttons.
See the official documentation: https://docs.oracle.com/javase/8/javase-clienttechnologies.htm