We will show you how to display an Image with JavaFX by selecting the image file with a button in the interface and displaying it in a VBox container.
In this example we use as a reference another previous one that we already did, but in a version of Java 8 and some things have changed for Java.
In our examples we use NetBeans and you may have encountered the following error:
Error: JavaFX runtime components are missing, and are required to run this application Command execution failed
This error appears because in recent versions of Java it is necessary to create modules and we must initialize the JavaFX conditions before running our example, a new feature added as of Java 9. To avoid this step we will create two Java classes: Main. java and JavaFXImage.java.
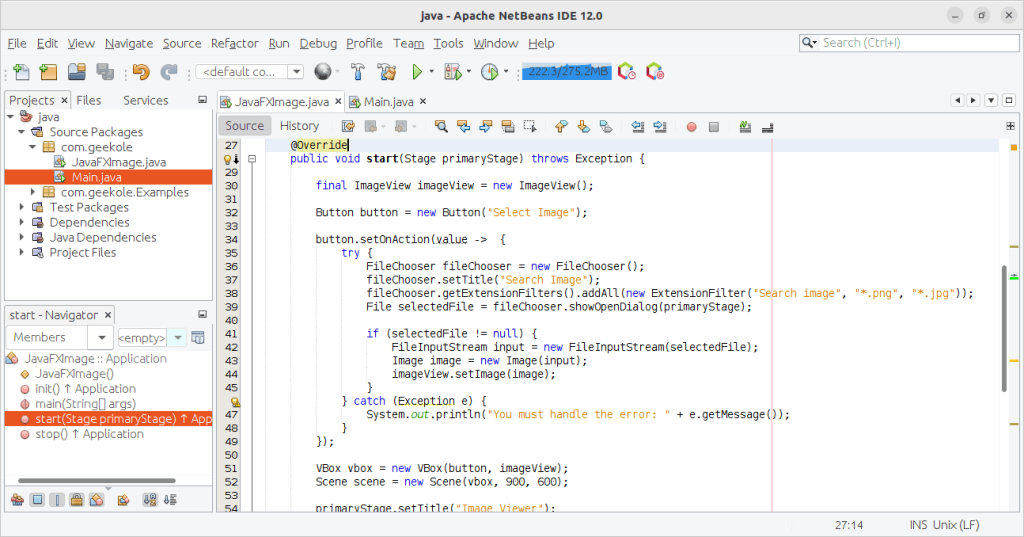
The code for Main.java is as follows:
package com.geekole; // Name of your java package /** * * @author geekole */ public class Main { public static void main(String[] args) { JavaFXImage.main(args); } }
Now our JavaFXImage.java class:
package com.geekole; // Name of your java package import java.io.File; import java.io.FileInputStream; import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.image.Image; import javafx.scene.image.ImageView; import javafx.scene.layout.VBox; import javafx.stage.FileChooser; import javafx.stage.FileChooser.ExtensionFilter; import javafx.stage.Stage; /** * * @author geekole.com */ public class JavaFXImage extends Application { @Override public void init() throws Exception { super.init(); System.out.println("Perform any necessary initializations here."); } @Override public void start(Stage primaryStage) throws Exception { final ImageView imageView = new ImageView(); Button button = new Button("Select Image"); button.setOnAction(value -> { try { FileChooser fileChooser = new FileChooser(); fileChooser.setTitle("Search Image"); fileChooser.getExtensionFilters().addAll(new ExtensionFilter("Search image", "*.png", "*.jpg")); File selectedFile = fileChooser.showOpenDialog(primaryStage); if (selectedFile != null) { FileInputStream input = new FileInputStream(selectedFile); Image image = new Image(input); imageView.setImage(image); } } catch (Exception e) { System.out.println("You must handle the error: " + e.getMessage()); } }); VBox vbox = new VBox(button, imageView); Scene scene = new Scene(vbox, 900, 600); primaryStage.setTitle("Image Viewer"); primaryStage.setScene(scene); primaryStage.show(); } @Override public void stop() throws Exception { super.stop(); System.out.println("Destroy the resources. Clean up."); } public static void main(String[] args) { launch(args); } }
A brief explanation of the code, the following lines instantiate our button and the component that will display the image:
final ImageView imageView = new ImageView(); Button button = new Button("Select image");
We now create a function to listen to events on the button and launch a FileChooser to which we add a filter with the image extensions that we want to display:
button.setOnAction(value -> { try { FileChooser fileChooser = new FileChooser(); fileChooser.setTitle("Search Image"); fileChooser.getExtensionFilters().addAll(new ExtensionFilter("Image Files", "*.png", "*.jpg")); File selectedFile = fileChooser.showOpenDialog(primaryStage); if (selectedFile != null) { FileInputStream input = new FileInputStream(selectedFile); Image image = new Image(input); imageView.setImage(image); } } catch (Exception e) { System.out.println("You should handle the error: " + e.getMessage()); } });
Lastly, we create a container that we add to a scene and then to the main stage. Finally we show it as a window:
VBox vbox = new VBox(button, imageView); Scene scene = new Scene(vbox, 900, 600); primaryStage.setTitle("Image Viewer"); primaryStage.setScene(scene); primaryStage.show();
Running as «executable file» from NetBeans to Main.java will bring up our window with a single element, our selection button:
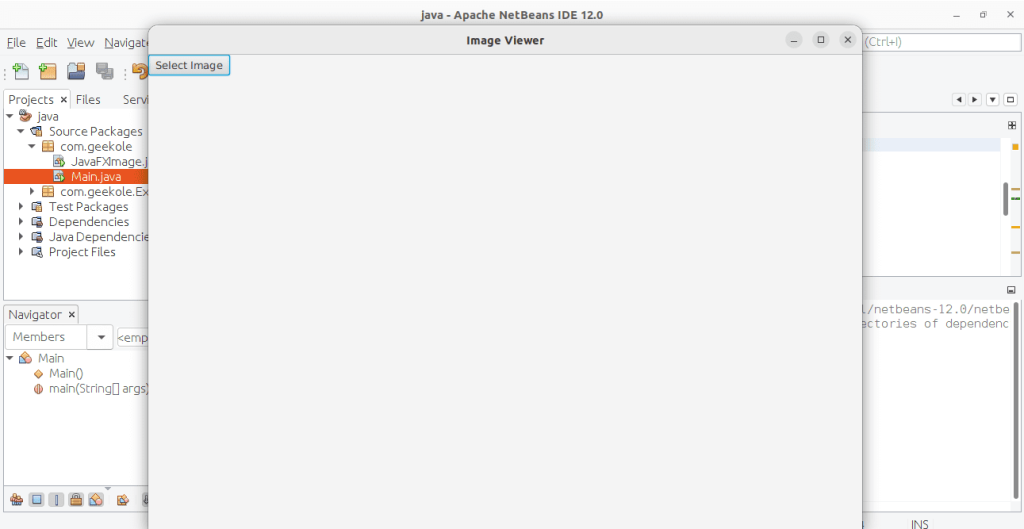
By clicking on the button, the file selection window will appear, the appearance of which will depend on the operating system in which you are working:
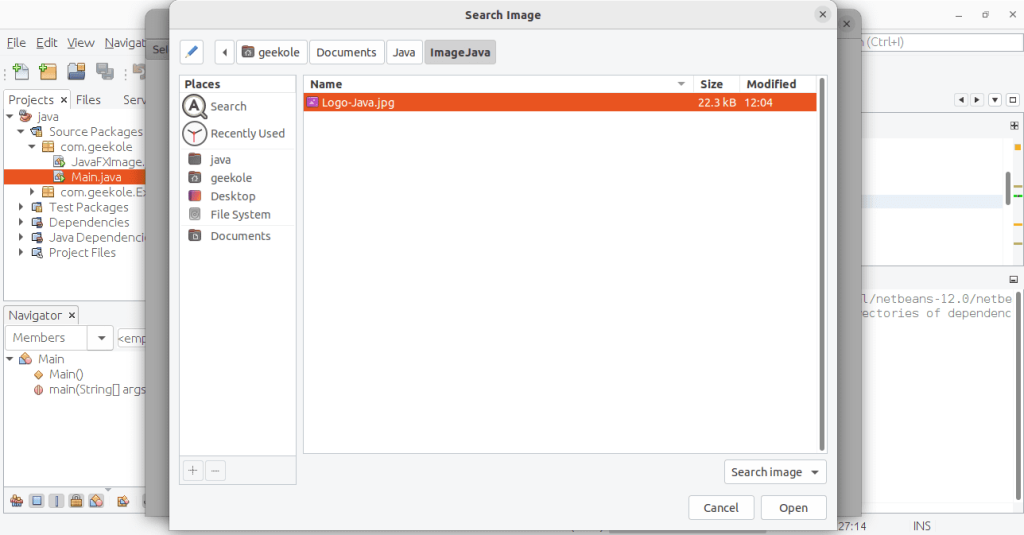
Our image will appear unfolded after you select the file and click «Open».
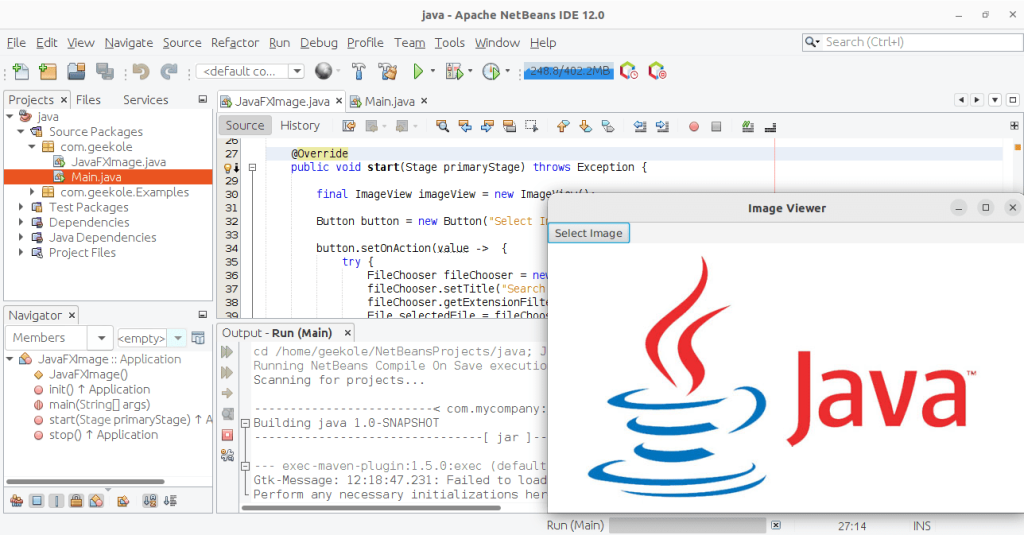
This example to Display an Image with JavaFX is simple, to start and understand how JavaFX works and it will help you.
See documentation: https://docs.oracle.com/javase/8/javafx/api/toc.htm
More: https://openjfx.io/