Read XML files in Java by this method is relatively simple but expensive due to the use of memory and execution time.
The file that we will use for reading in this example is the following:
data.xml
<?xml version="1.0"?> <company> <employee id="1"> <name>Jackson Aiden</name> <username>jackson</username> <password>321423</password> </employee> <employee id="2"> <name>Emma Sophia</name> <username>emma</username> <password>433543</password> </employee> </company>
We will use the dom libraries from the Maven repository.
<dependency> <groupId>org.w3c</groupId> <artifactId>dom</artifactId> <version>2.3.0-jaxb-1.0.6</version> </dependency>
Finally the code.
package com.geekole; // Name of your java package import java.io.File; import javax.xml.parsers.DocumentBuilder; import javax.xml.parsers.DocumentBuilderFactory; import org.w3c.dom.Document; import org.w3c.dom.Element; import org.w3c.dom.Node; import org.w3c.dom.NodeList; /** * * @author geekole */ public class ReadXML { public static void main(String args[]) { try { File file = new File("/path/data.xml"); DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance(); DocumentBuilder documentBuilder = dbf.newDocumentBuilder(); Document document = documentBuilder.parse(file); document.getDocumentElement().normalize(); System.out.println("Root element:" + document.getDocumentElement().getNodeName()); NodeList EmployeeList = document.getElementsByTagName("employee"); for (int temp = 0; temp < EmployeeList.getLength(); temp++) { Node node = EmployeeList.item(temp); System.out.println("Element:" + node.getNodeName()); if (node.getNodeType() == Node.ELEMENT_NODE) { Element element = (Element) node; System.out.println("id: " + element.getAttribute("id")); System.out.println("Name: " + element.getElementsByTagName("name").item(0).getTextContent()); System.out.println("username: " + element.getElementsByTagName("username").item(0).getTextContent()); System.out.println("password: " + element.getElementsByTagName("password").item(0).getTextContent()); } } } catch (Exception e) { e.printStackTrace(); } } }
As you can see DocumentBuilder allows us to parse the XML file.
The function document.getDocumentElement().normalize(); it gives the text a more pleasing visual format but it is not essential.
The NodeList EmployeeList = document.getElementsByTagName(“employee”) function; it is basically to obtain the list of employee nodes that come in our XML file. It is necessary to know the structure of the XML nodes that come in the file to extract specific information. After reading each used employee node it is possible to get its attributes using element.getAttribute(“id”) or if necessary the text content of a particular node as in element.getElementsByTagName(“name”).item(0). getTextContent().
Example of Read XML files in Java
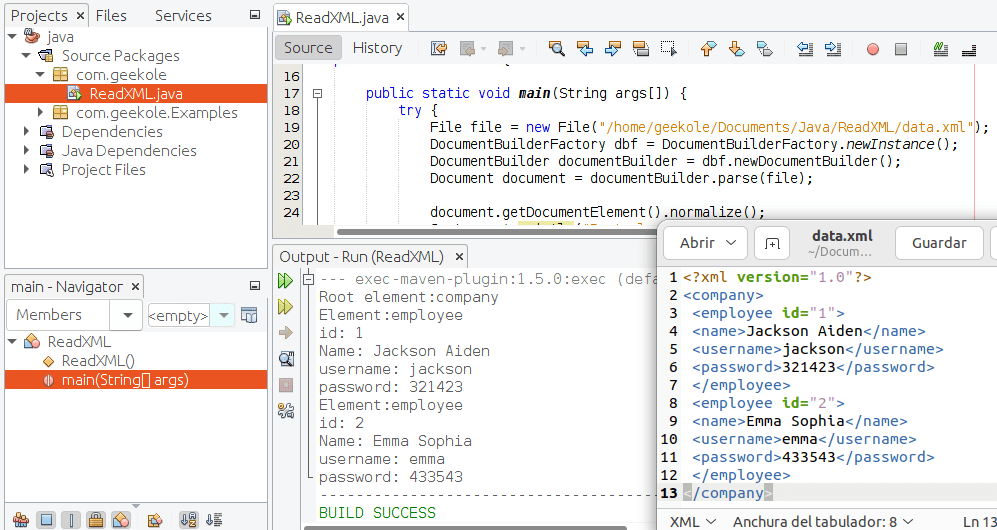
There are other reading methods that we will see soon.
Update: A more efficient but more difficult way to implement is the one we show you in this post: