Sometimes the structure of an IF statement can take several lines of code and do very little. In Java the Ternary Conditional Operator ? which can replace an if and multiple lines of code.
You may also like:
For example, in the following lines of code:
package com.geekole; // Name of your java package public class IFExample { public static void main(String args[]) { int i = 1; int j = 2; int majorNumber = 0; if (i > j) { majorNumber = i; } else { majorNumber = j; } System.out.println("majorNumber: " + majorNumber); } }
The structure of the if statement takes us four lines, of course you can put everything on one line, but we are still using some code.
This statement can be changed to a more condensed form of code with the help of the Ternary Operator ? which has the following form:
result = (condition)? firstValue : secondValue;
If you look at the structure, in the first part of the statement after the assignment, we evaluate a condition, in the second we assign a value firstValue to the result variable if the condition is true, or secondValue if the condition is false.
So our previous if example would look like the following code:
package com.geekole; // Name of your java package public class IFExample { public static void main(String args[]) { int i = 1; int j = 2; int majorNumber = 0; majorNumber = i > j ? i : j; System.out.println("majorNumber: " + majorNumber); } }
The result in the console of executing the above code will be the following:
majorNumber: 2
We have saved at least four lines of code. If you notice, unlike the if statement, we are assigning a value to a variable directly and as a result of evaluating a condition. With the if, we have to do the assignment explicitly. Another thing to note is that when the condition is true, the value of i is assigned, which is separated by a colon, from the assignment of the second value j, which should be done in case the condition is false.
So that you notice that this operator can be useful, you have the following example:
package com.geekole; // Name of your java package public class IFExample { public static void main(String args[]) { int i = 1; int j = 2; System.out.println("Major Number: " + ((i > j) ? "i is greater" : "j is greater")); } }
The result in console:
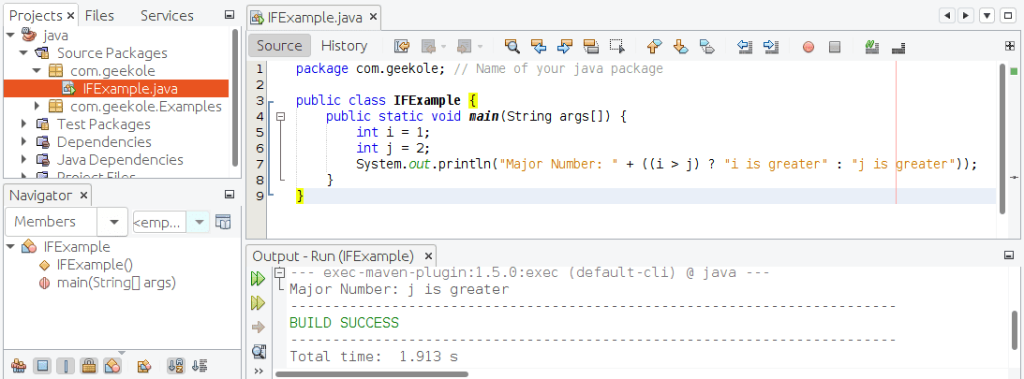
If you notice, we have not only avoided using an IF statement, we have also used the operator to evaluate and concatenate with another string the result of our evaluation and assignment of a string within another statement. If the condition is met, “majorNumber: “ will be concatenated to the string “i is greater”, otherwise, “j is greater” will be concatenated. The console output for this example is:
majorNumber: j is greater
The code is much more compact when using the Ternary Operator ?
However, many programmers, even knowing how useful this operator is, prefer to use if statements, to make the code easy to read. It is a matter of taste and style.
We hope you find this brief explanation of the ternary operator in Java useful.
See documentation: https://docs.oracle.com/javase/tutorial/java/nutsandbolts/op2.html