One of the most used structures in the world of programming is the for loop. Of course Java handles it and there are variants of this statement that can be used according to specific needs.
You may also like:
This is the most popular for loop structure:
for (initialization; terminación; increment) { statements }
This statement for the for loop uses three components:
In the initialization, the variables that will be used to control the number of times that the statements inside the code block will be executed are declared.
The termination block sets the boolean condition for the statements within the loop code block to be executed.
In the increment, the value of the variables declared in the initialization is modified and it continues modifying its value until the termination statement is fulfilled.
Between the brackets are the code statements, which can be any code and often include the values of the initialized variables that change with each cycle.
Here’s an example of the for loop that you can compile and test:
package com.geekole; // Name of your java package /** * * @author geekole.com */ public class ForLoop { public static void main(String args[]){ for (int i = 0; i < 10; i++) { System.out.println("i: " + i); } } }
In the previous example, the variable “i” is assigned the value zero, in the termination statement while “i” is less than 10, the for loop will execute the statement contained between the brackets. In the increment block “i” it increases its value by one after each cycle using the “++” operator.
If you run the code:
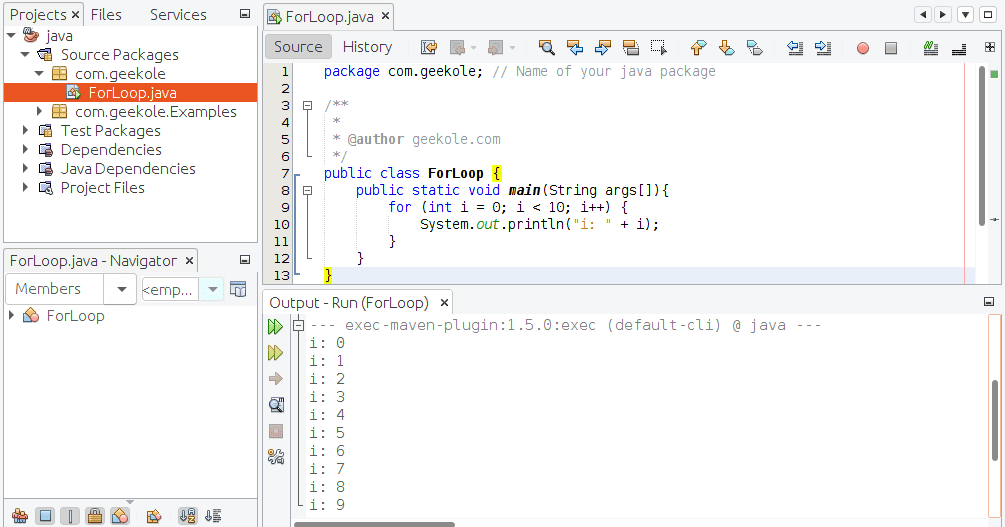
What you get is a console listing from 0 to 9.
It is also possible to do a descending version of printing numbers
package com.geekole; // Name of your java package /** * * @author geekole.com */ public class ForLoop2 { public static void main(String args[]){ for (int i = 10; i > 0; i--) { System.out.println("i: " + i); } } }
The result is as follows:
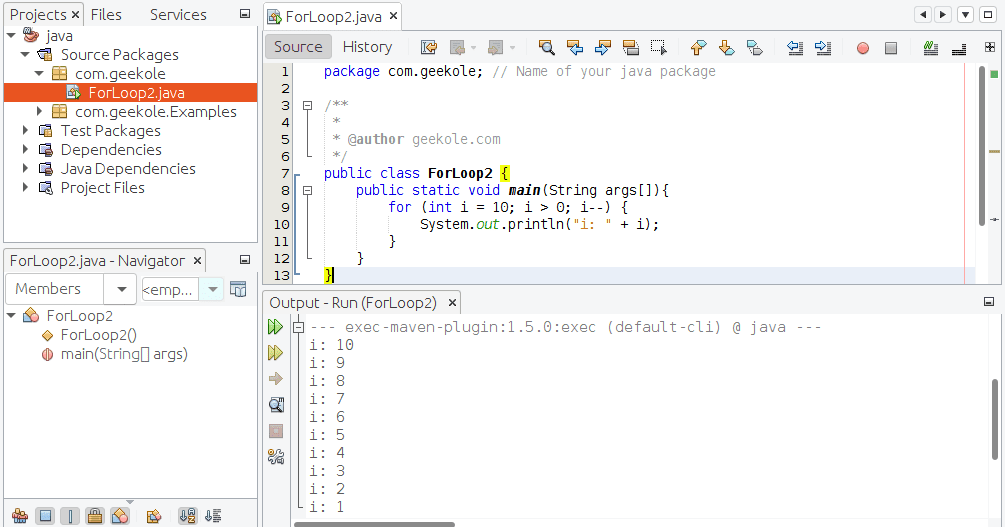
Another variant that is possible with the for loop is the one that allows you to execute the statements indefinitely, skipping initialization, termination, and increment.
package com.geekole; // Name of your java package /** * * @author geekole.com */ public class ForLoop3 { public static void main(String args[]){ for (;;) { System.out.println("Hello"); } } }
We get a loop of code that never ends.
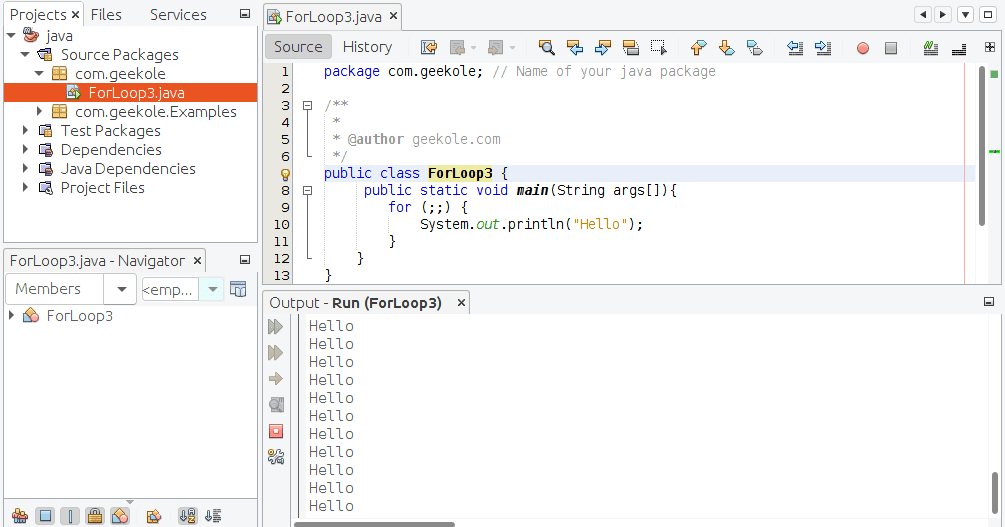
In recent versions of Java, a condensed form of the for loop has been added that was created to make it easier to handle Collections and Arrays.
package com.geekole; // Name of your java package /** * * @author geekole.com */ public class ForLoop4 { public static void main(String args[]){ int[] numbers = {1,2,3,4,5,6,7,8,9,10}; for (int number : numbers) { System.out.println("Value: " + number); } } }
In this version you only have to declare the name of the variable with its type to which the value or reference is to be assigned (int number) and after the colon the reference to the array or collection (numbers). It’s an elegant and more compact way of implementing the for loop that works in an infinite number of circumstances.
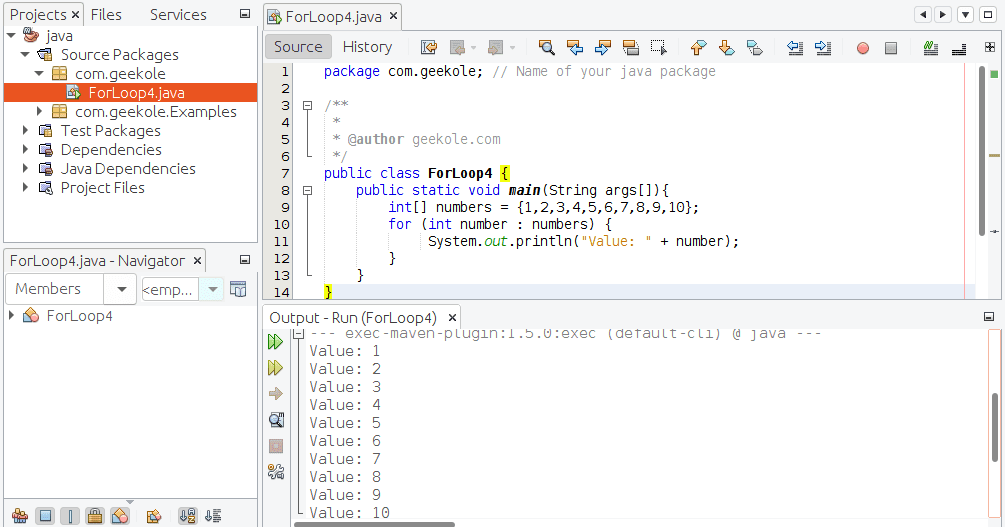
We hope these examples of the for loop in Java will help you.