One of the easiest ways to create a Window, GUI graphical interfaces in Python is with the help of tkinter.
GUIs often use an event-driven form of OO programming, the program responds to events, which are actions that a user performs. The actions that the user performs are nothing more than buttons that are pressed.
You may also like:
# geekole.com from tkinter import Tk, Label, Button class ExampleWindow: def __init__(self, master): self.master = master master.title("A simple graphical interface") self.label = Label(master, text="This is the first window!") self.label.pack() self.greetingButton = Button(master, text="Greet", command=self.greet) self.greetingButton.pack() self.closeButton = Button(master, text="Close", command=master.quit) self.closeButton.pack() def greet(self): print("¡Hey!") root = Tk() myWindow = ExampleWindow(root) root.mainloop()
In the code, you can notice that the greetingButton and closeButton buttons are assigned to a specific action, the former calls the “greet” function when pressed, the latter closes our window.
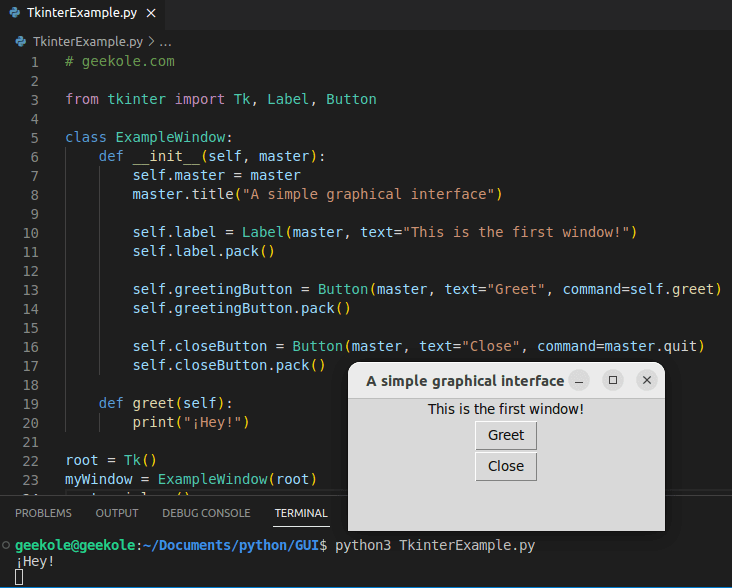
When the button labeled “Greet” is pressed, the following message is printed on the console:
¡Hey!
We hope this example of how to create a Window or GUI in Python will be useful to you.