We will show you how to create text files in Java with two different techniques.
You may also like:
– Read a text file – Create an XML file – How to create a ZIP file – Create an Excel file – How to create a PDF |
Create files using FileWriter and BufferedWriter
The first strategy is using the FileWriter and BufferedWriter classes, where we use the write method that allows you to write strings or arrays of characters.
The second way is using PrintWriter which allows you to do more or less the same thing, but in a more summarized way and with the possibility of writing other types of data on the file.
package com.geekole; // Name of your java package import java.io.BufferedWriter; import java.io.File; import java.io.FileWriter; /** * @author geekole.com */ public class ExampleCreateFile { public static void main(String ars[]){ try { String path = "/path/filename.txt"; String content = "Sample content"; File file = new File(path); // If the file does not exist it is created if (!file.exists()) { file.createNewFile(); } FileWriter fw = new FileWriter(file); BufferedWriter bw = new BufferedWriter(fw); bw.write(content); bw.close(); } catch (Exception e) { e.printStackTrace(); } } }
The writing is done in these statements:
FileWriter fw = new FileWriter(file); BufferedWriter bw = new BufferedWriter(fw); bw.write(content); bw.close();
The FileWriter class must be created with a reference to a File class that contains the details of the file to be created.
The content of the text is created with the function bw.write(content) from BufferedWriter and it will be up to you to add the newline character.
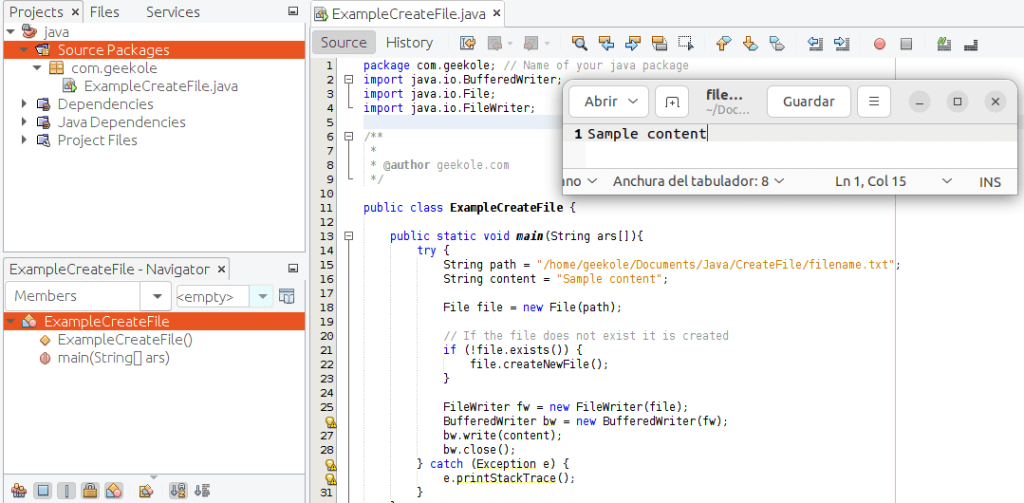
Create files using PrintWriter
The code is the following:
import java.io.PrintWriter; public class CreateFile { public static void main(String ars[]){ try { PrintWriter writer = new PrintWriter("/path/filename2.txt", "UTF-8"); writer.println("First line"); writer.println("Second line"); writer.close(); } catch (Exception e) { e.printStackTrace(); } } }
In this second option, the PrintWriter class can receive a string with the path of the file or an instance of File, the second argument is the type of encoding to be used (“UTF-8”), which will be useful if you need to save text with special characters. The writing work is done with the println method, which allows you to write other data types such as integers, floating point, booleans, and characters.
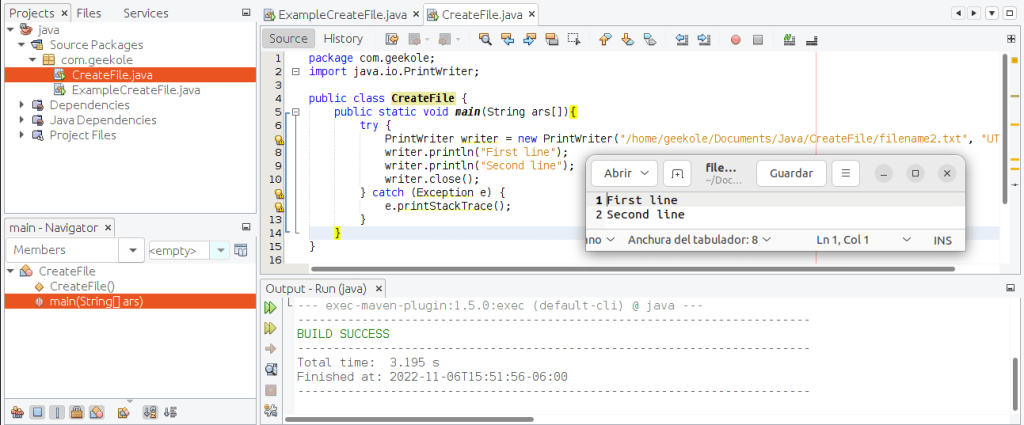
Create text files in Java does not require many lines of code. We hope that the examples will be useful to you.
See documentation: https://docs.oracle.com/javase/7/docs/api/java/io/BufferedWriter.html