We show you the code to get information from a file in Java, with the help of the File class. The File class is in the java.io package that comes as part of the standard Java packages.
We show you the code:
package com.geekole; // Name of your java package import java.io.File; /** * @author geekole.com */ public class GetInformationFile { public static void main(String[] args) { File file = new File("/path/filename.txt"); if (file.exists()) { System.out.println("Filename: " + file.getName()); System.out.println("Absolute path: " + file.getAbsolutePath()); System.out.println("Write permissions: " + file.canWrite()); System.out.println("Read permissions: " + file.canRead()); System.out.println("File size: " + file.length()); System.out.println("Container folder: " + file.getParent()); System.out.println("Is file: " + file.isFile()); System.out.println("Is directory " + file.isDirectory()); System.out.println("Is hidden: " + file.isHidden()); } else { System.out.println("The file does not exists."); } } }
In the first few lines after instantiating the File class with the file path, we first check that the file exists with the exist() method. This is a common practice that you should always consider when handling files.
if (file.exists()) { . } else { System.out.println("The file does not exists."); }
We get the name and path of the file, in case we need to know it later:
System.out.println("Filename: " + file.getName()); System.out.println("Absolute path: " + file.getAbsolutePath());
We verify if the file has read or write permissions, this information is important because not having permissions is likely to generate an exception during file handling:
System.out.println("Write permissions: " + file.canWrite()); System.out.println("Read permissions: " + file.canRead());
And some other functions like knowing the name of the parent folder, if it is a file, if it is a directory or if it is a hidden file:
System.out.println("Container folder: " + file.getParent()); System.out.println("Is file: " + file.isFile()); System.out.println("Is directory " + file.isDirectory()); System.out.println("Is hidden: " + file.isHidden());
When compiling and executing the code we will obtain the information of the file, in our case we use Netbeans to test the example:
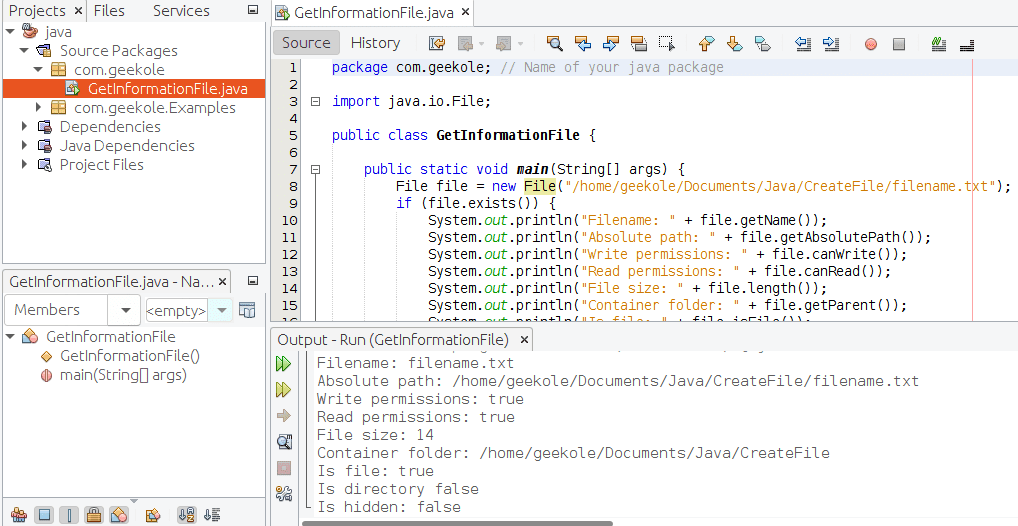
We hope that this example to obtain information from a file in Java will be useful to you, we also leave you the links to create or read text files:
See more information: https://docs.oracle.com/javase/7/docs/api/java/io/File.html